实现一段tensorflow2.0 LSTM文本分类的代码,使用glove进行词嵌入,并给出示例
时间: 2023-05-20 08:05:27 浏览: 126
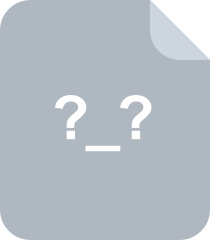
Tensorflow中LSTM使用方法示例

以下是一段使用TensorFlow 2.0实现的LSTM文本分类代码,使用GloVe进行词嵌入的示例:
```python
import tensorflow as tf
import numpy as np
# 加载GloVe词向量
glove_file = 'glove.6B.100d.txt'
embedding_index = {}
with open(glove_file, encoding='utf-8') as f:
for line in f:
values = line.split()
word = values[0]
coefs = np.asarray(values[1:], dtype='float32')
embedding_index[word] = coefs
# 构建词向量矩阵
embedding_dim = 100
max_words = 10000
embedding_matrix = np.zeros((max_words, embedding_dim))
for word, i in tokenizer.word_index.items():
if i < max_words:
embedding_vector = embedding_index.get(word)
if embedding_vector is not None:
embedding_matrix[i] = embedding_vector
# 构建模型
model = tf.keras.Sequential([
tf.keras.layers.Embedding(max_words, embedding_dim, input_length=maxlen),
tf.keras.layers.LSTM(32),
tf.keras.layers.Dense(1, activation='sigmoid')
])
# 编译模型
model.layers[0].set_weights([embedding_matrix])
model.layers[0].trainable = False
model.compile(optimizer='rmsprop', loss='binary_crossentropy', metrics=['acc'])
# 训练模型
history = model.fit(x_train, y_train, epochs=10, batch_size=32, validation_data=(x_val, y_val))
```
其中,`glove.6B.100d.txt`是GloVe预训练的100维词向量文件,可以从GloVe官网下载。`max_words`是词汇表的大小,`maxlen`是输入序列的最大长度。在构建词向量矩阵时,只使用了词汇表中前`max_words`个词的词向量,其余词的词向量均为0。在构建模型时,首先使用`Embedding`层加载词向量矩阵,然后使用`LSTM`层进行序列建模,最后使用`Dense`层输出二分类结果。在编译模型时,将词向量矩阵的权重设置为不可训练,以避免过拟合。在训练模型时,使用`fit`方法进行训练,其中`x_train`和`y_train`是训练集的输入和输出,`x_val`和`y_val`是验证集的输入和输出。
阅读全文
相关推荐
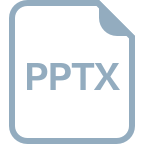
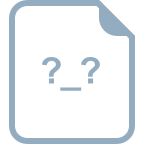
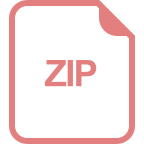
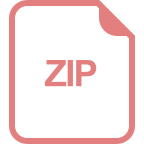
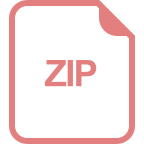
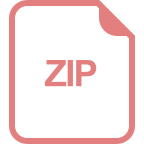
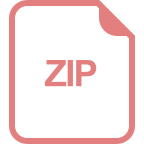
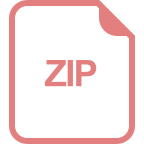
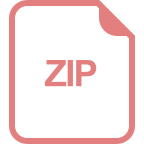
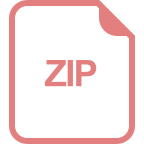
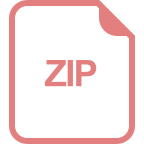
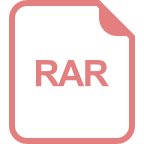
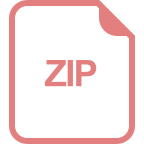
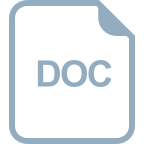
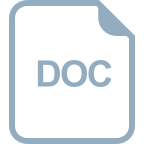