java停车场管理系统代码
时间: 2023-07-31 21:05:21 浏览: 54
很抱歉,我作为一个AI语言模型,没有办法提供完整的停车场管理系统代码。但是,我可以给你一些实现停车场管理系统的思路和建议。
停车场管理系统一般包括以下几个模块:
1. 停车场信息管理模块:包括停车场的基本信息,车位数量,收费标准等信息。
2. 车辆信息管理模块:包括车辆的基本信息,车辆类型,入场时间,离场时间等信息。
3. 车位管理模块:实现车位的分配和释放功能。
4. 收费管理模块:实现车辆停车费用的计算和收取。
下面是一个简单的停车场管理系统的Java代码示例,仅供参考:
```
import java.util.*;
class ParkingLot {
private int capacity; // 停车场总车位数
private int available; // 剩余可用车位数
private double hourlyRate; // 每小时收费
private Map<String, Car> parkedCars; // 存储已停车辆的信息
public ParkingLot(int capacity, double hourlyRate) {
this.capacity = capacity;
this.available = capacity;
this.hourlyRate = hourlyRate;
this.parkedCars = new HashMap<>();
}
public boolean isFull() {
return available == 0;
}
public double getHourlyRate() {
return hourlyRate;
}
public int getAvailable() {
return available;
}
public int getCapacity() {
return capacity;
}
public boolean parkCar(Car car) {
if (isFull()) {
return false;
}
parkedCars.put(car.getLicensePlate(), car);
available--;
return true;
}
public Car removeCar(String licensePlate) {
Car car = parkedCars.remove(licensePlate);
if (car != null) {
available++;
}
return car;
}
public double calculateFee(Car car) {
long duration = car.getParkingDuration();
double fee = hourlyRate * Math.ceil(duration / 3600.0);
return fee;
}
}
class Car {
private String licensePlate; // 车牌号
private String type; // 车型
private long enterTime; // 入场时间
public Car(String licensePlate, String type) {
this.licensePlate = licensePlate;
this.type = type;
this.enterTime = System.currentTimeMillis() / 1000;
}
public String getLicensePlate() {
return licensePlate;
}
public String getType() {
return type;
}
public long getEnterTime() {
return enterTime;
}
public long getParkingDuration() {
long now = System.currentTimeMillis() / 1000;
return now - enterTime;
}
}
public class ParkingLotSystem {
public static void main(String[] args) {
ParkingLot parkingLot = new ParkingLot(50, 5.0);
Car car1 = new Car("粤A12345", "小汽车");
Car car2 = new Car("粤B67890", "大巴车");
if (parkingLot.parkCar(car1)) {
System.out.println("车辆 " + car1.getLicensePlate() + " 停车成功!");
} else {
System.out.println("车位已满,停车失败!");
}
if (parkingLot.parkCar(car2)) {
System.out.println("车辆 " + car2.getLicensePlate() + " 停车成功!");
} else {
System.out.println("车位已满,停车失败!");
}
Car car3 = parkingLot.removeCar("粤A12345");
if (car3 != null) {
double fee = parkingLot.calculateFee(car3);
System.out.println("车辆 " + car3.getLicensePlate() + " 离开停车场,停车费用为 " + fee + " 元。");
} else {
System.out.println("未找到指定车辆!");
}
}
}
```
这段代码实现了一个简单的停车场管理系统,包括停车场信息管理、车辆信息管理、车位管理和收费管理等功能。你可以根据自己的需求,进行修改和扩展。
相关推荐
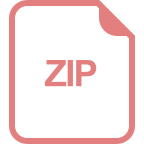
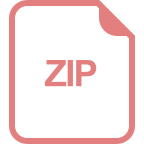
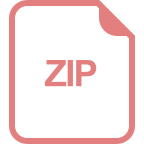














