用菜单+CardLayout 布局,通过录入界面将多人的通讯录写入文件“通讯录.txt”,点击“显示”菜单项,显示通讯录
时间: 2023-06-16 10:02:31 浏览: 73
好的,这里是一个基于菜单+CardLayout布局的Java通讯录程序示例,可以将多人的通讯录写入文件并读取并显示通讯录。
```java
import java.awt.*;
import java.awt.event.*;
import java.io.*;
import javax.swing.*;
public class AddressBook extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private CardLayout cardLayout;
private JPanel cardPanel;
private JTextArea displayArea;
private JTextField nameField, phoneField, emailField;
private JButton addButton, saveButton;
public AddressBook() {
super("Address Book");
// Create menu bar
JMenuBar menuBar = new JMenuBar();
setJMenuBar(menuBar);
// Create "File" menu
JMenu fileMenu = new JMenu("File");
menuBar.add(fileMenu);
// Create "Add" menu item
JMenuItem addMenuItem = new JMenuItem("Add");
addMenuItem.addActionListener(this);
fileMenu.add(addMenuItem);
// Create "Save" menu item
JMenuItem saveMenuItem = new JMenuItem("Save");
saveMenuItem.addActionListener(this);
fileMenu.add(saveMenuItem);
// Create "Display" menu item
JMenuItem displayMenuItem = new JMenuItem("Display");
displayMenuItem.addActionListener(this);
fileMenu.add(displayMenuItem);
// Create card panel
cardLayout = new CardLayout();
cardPanel = new JPanel(cardLayout);
getContentPane().add(cardPanel);
// Create "Add" panel
JPanel addPanel = new JPanel(new GridLayout(3, 2));
addPanel.add(new JLabel("Name:"));
nameField = new JTextField();
addPanel.add(nameField);
addPanel.add(new JLabel("Phone:"));
phoneField = new JTextField();
addPanel.add(phoneField);
addPanel.add(new JLabel("Email:"));
emailField = new JTextField();
addPanel.add(emailField);
addButton = new JButton("Add");
addButton.addActionListener(this);
addPanel.add(addButton);
cardPanel.add(addPanel, "ADD");
// Create "Display" panel
JPanel displayPanel = new JPanel(new BorderLayout());
displayArea = new JTextArea();
JScrollPane scrollPane = new JScrollPane(displayArea);
displayPanel.add(scrollPane, BorderLayout.CENTER);
cardPanel.add(displayPanel, "DISPLAY");
// Set default panel
cardLayout.show(cardPanel, "ADD");
// Set frame properties
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(400, 300);
setLocationRelativeTo(null);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == addButton) {
// Add contact to file
String name = nameField.getText().trim();
String phone = phoneField.getText().trim();
String email = emailField.getText().trim();
if (!name.isEmpty() && !phone.isEmpty() && !email.isEmpty()) {
try {
FileWriter fileWriter = new FileWriter("通讯录.txt", true);
fileWriter.write(name + "," + phone + "," + email + "\n");
fileWriter.close();
JOptionPane.showMessageDialog(this, "Contact added.");
nameField.setText("");
phoneField.setText("");
emailField.setText("");
} catch (IOException ex) {
JOptionPane.showMessageDialog(this, "Error: " + ex.getMessage(), "Error", JOptionPane.ERROR_MESSAGE);
}
} else {
JOptionPane.showMessageDialog(this, "Please fill in all fields.", "Error", JOptionPane.ERROR_MESSAGE);
}
} else if (e.getSource() == saveButton) {
// Save contacts to file
try {
FileWriter fileWriter = new FileWriter("通讯录.txt", false);
fileWriter.write(displayArea.getText());
fileWriter.close();
JOptionPane.showMessageDialog(this, "Contacts saved.");
} catch (IOException ex) {
JOptionPane.showMessageDialog(this, "Error: " + ex.getMessage(), "Error", JOptionPane.ERROR_MESSAGE);
}
} else if (e.getActionCommand().equals("Display")) {
// Display contacts in text area
try {
BufferedReader bufferedReader = new BufferedReader(new FileReader("通讯录.txt"));
String line;
StringBuilder stringBuilder = new StringBuilder();
while ((line = bufferedReader.readLine()) != null) {
String[] parts = line.split(",");
stringBuilder.append("Name: ").append(parts[0]).append("\n");
stringBuilder.append("Phone: ").append(parts[1]).append("\n");
stringBuilder.append("Email: ").append(parts[2]).append("\n\n");
}
bufferedReader.close();
displayArea.setText(stringBuilder.toString());
cardLayout.show(cardPanel, "DISPLAY");
} catch (IOException ex) {
JOptionPane.showMessageDialog(this, "Error: " + ex.getMessage(), "Error", JOptionPane.ERROR_MESSAGE);
}
} else if (e.getActionCommand().equals("Add")) {
// Switch to add panel
cardLayout.show(cardPanel, "ADD");
} else if (e.getActionCommand().equals("Save")) {
// Switch to save panel
saveButton = new JButton("Save");
saveButton.addActionListener(this);
JPanel savePanel = new JPanel(new BorderLayout());
savePanel.add(new JScrollPane(displayArea), BorderLayout.CENTER);
savePanel.add(saveButton, BorderLayout.SOUTH);
cardPanel.add(savePanel, "SAVE");
cardLayout.show(cardPanel, "SAVE");
}
}
public static void main(String[] args) {
new AddressBook();
}
}
```
在该程序中,我们使用了JFrame、JMenuBar、JMenu、JMenuItem、CardLayout、JPanel、JTextArea、JTextField和JButton等Swing组件。其中,JMenuBar、JMenu和JMenuItem用于创建菜单栏和菜单项,CardLayout和JPanel用于实现多个面板的切换,JTextArea、JTextField和JButton用于录入、显示和保存通讯录信息。
在程序中,我们首先创建了菜单栏和菜单项,并将它们添加到窗口的顶部。然后,我们创建了一个CardLayout布局的JPanel,并在其中添加了两个面板:录入面板和显示面板。录入面板包含三个文本框和一个“添加”按钮,用于录入通讯录信息并将其写入文件。显示面板包含一个文本区域,用于显示从文件中读取的通讯录信息。我们使用了CardLayout布局,使得录入面板和显示面板可以在需要时进行切换。当用户点击“显示”菜单项时,程序会读取文件中的通讯录信息,并在文本区域中显示它们。当用户点击“添加”菜单项时,程序会切换到录入面板,以便用户可以录入新的通讯录信息。当用户点击“保存”菜单项时,程序会切换到一个新的面板,该面板包含一个文本区域和一个“保存”按钮,用于编辑并保存通讯录信息。
请注意,在该程序中,我们将通讯录信息保存在名为“通讯录.txt”的文件中。如果您想要将文件保存在不同的位置或使用不同的文件名,请相应地更改代码。
相关推荐
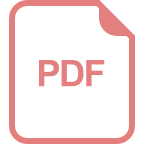














