html页面,回显部门树
时间: 2023-07-25 08:42:20 浏览: 87
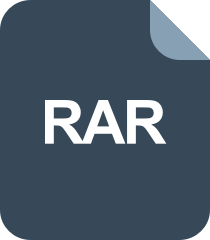
h5移动端部门树展示
如果你想在 HTML 页面上回显部门树,可以使用 HTML 的 `<ul>` 和 `<li>` 标签组合来实现。具体步骤如下:
1. 构造部门树的数据结构,例如使用一个数组或者对象来表示每个部门的信息,包括部门名称、子部门等。
```
var departmentTree = [
{
name: '部门A',
children: [
{
name: '部门A-1',
children: []
},
{
name: '部门A-2',
children: [
{
name: '部门A-2-1',
children: []
},
{
name: '部门A-2-2',
children: []
}
]
}
]
},
{
name: '部门B',
children: [
{
name: '部门B-1',
children: []
},
{
name: '部门B-2',
children: [
{
name: '部门B-2-1',
children: []
},
{
name: '部门B-2-2',
children: []
}
]
}
]
}
];
```
2. 在 HTML 页面中使用 `<ul>` 和 `<li>` 标签组合来展示部门树,可以使用递归函数来处理部门树的嵌套结构。例如:
```
<!DOCTYPE html>
<html>
<head>
<title>部门树</title>
<meta charset="UTF-8">
</head>
<body>
<ul id="departmentTree"></ul>
<script>
var departmentTree = [
{
name: '部门A',
children: [
{
name: '部门A-1',
children: []
},
{
name: '部门A-2',
children: [
{
name: '部门A-2-1',
children: []
},
{
name: '部门A-2-2',
children: []
}
]
}
]
},
{
name: '部门B',
children: [
{
name: '部门B-1',
children: []
},
{
name: '部门B-2',
children: [
{
name: '部门B-2-1',
children: []
},
{
name: '部门B-2-2',
children: []
}
]
}
]
}
];
function renderDepartmentTree(departmentTree, ulElement) {
departmentTree.forEach(function(department) {
var liElement = document.createElement('li');
liElement.innerText = department.name;
if (department.children.length > 0) {
var subUlElement = document.createElement('ul');
liElement.appendChild(subUlElement);
renderDepartmentTree(department.children, subUlElement);
}
ulElement.appendChild(liElement);
});
}
var departmentTreeElement = document.getElementById('departmentTree');
renderDepartmentTree(departmentTree, departmentTreeElement);
</script>
</body>
</html>
```
在上面的示例代码中,我们使用了递归函数 `renderDepartmentTree` 来遍历部门树的嵌套结构,根据每个部门的信息生成一个 `<li>` 元素,并将其添加到 `<ul>` 元素中。如果当前部门还有子部门,就递归调用 `renderDepartmentTree` 函数来处理子部门。最后,我们将生成的部门树添加到 HTML 页面中的 `<ul>` 元素中。
阅读全文
相关推荐
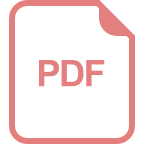
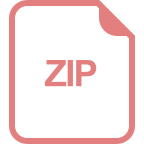











