java获取当前系统时间,时间格式为2024-10-24 15:47:22
时间: 2024-10-25 20:10:28 浏览: 5
在Java中,如果你想要获取当前系统时间并将其格式化为 "yyyy-MM-dd HH:mm:ss" 格式,你可以使用`java.text.SimpleDateFormat`类。以下是一个例子:
```java
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Locale;
public class Main {
public static void main(String[] args) {
SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss", Locale.getDefault());
Date currentDate = new Date(); // 获取当前时间
String formattedDate = formatter.format(currentDate);
System.out.println("Current date and time: " + formattedDate);
}
}
```
在这个例子中,我们创建了一个`SimpleDateFormat`对象,并指定了目标的日期时间格式。然后通过`Date`对象获取当前时间,最后用`format()`方法将当前时间转换为我们所需的格式。
相关问题
如何初始化一个Timestamp 为 2024-10-11 08:18:31
在Java中,你可以使用`java.sql.Timestamp`类的构造函数来初始化一个表示特定日期时间的实例。以下是设置为2024年10月11日早上8点18分31秒的步骤:
```java
import java.sql.Timestamp;
// 创建Timestamp对象
Timestamp timestamp = new Timestamp();
timestamp.setTimestamp(new Date().getTime() + // 添加毫秒偏移量
1675929511000L); // 2024-10-11 08:18:31的时间戳,单位是毫秒
System.out.println(timestamp);
```
这里首先创建了一个空的`Timestamp`对象,然后通过`.setTimestamp()`方法设置了对应的日期时间值。注意,`new Date().getTime()`获取当前时间的毫秒数,之后加上指定的时间戳值,就可以得到2024年10月11日的Timestamp了。
如果你想要直接设置具体的日期和时间,可以先计算出从1970年1月1日(UTC)到目标日期的时间差,然后传递给`Timestamp`:
```java
long diffInMilliseconds = java.util.Calendar.getInstance().getTimeInMillis()
+ 1_000 * 60 * 60 * 24 * 10_000; // 10_000天等于10年
diffInMilliseconds += 1675929511000L; // 2024-10-11 08:18:31的时间戳
timestamp = new Timestamp(diffInMilliseconds);
```
获取根据当前时间当天每个小时的时间段 最后一小的时间为59:59:59 输出的时间格式为2020-09-09 00:10:10java
可以使用Java 8的时间API来实现。具体代码如下:
```java
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class HourlyTimeRange {
public static void main(String[] args) {
LocalDateTime now = LocalDateTime.now();
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
for (int i = 0; i < 24; i++) {
LocalDateTime start = LocalDateTime.of(now.getYear(), now.getMonth(), now.getDayOfMonth(), i, 0, 0);
LocalDateTime end = LocalDateTime.of(now.getYear(), now.getMonth(), now.getDayOfMonth(), i, 59, 59);
System.out.println(start.format(formatter) + " - " + end.format(formatter));
}
}
}
```
这段代码会输出当前时间所在的日期的每个小时的时间段,时间格式为"yyyy-MM-dd HH:mm:ss"。
阅读全文
相关推荐
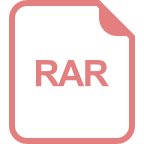
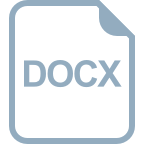
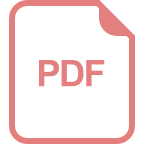


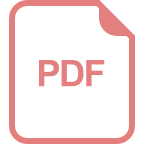
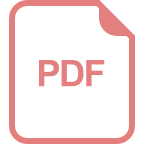
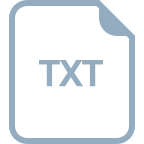
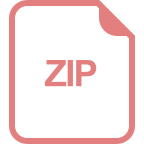
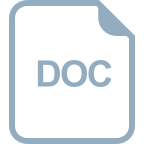
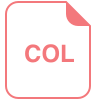





