uniapp+ts封装请求pinia配置pinia持久化及使用
时间: 2024-11-23 13:15:43 浏览: 86
UniApp结合TypeScript(简称TS)封装网络请求,并利用Vue.js的状态管理库Pinia进行数据管理和持久化,可以提高代码组织和维护效率。以下是基本步骤:
1. 安装依赖:
- 首先确保已经安装了`uni-app`, `vue3-persist-storage` 和 `@pinia/store` 等依赖。
```bash
npm install uni-app ts axios vue3-persist-storage @pinia/store
```
2. 创建Pinia store:
- 在`src/stores`目录下创建一个新的文件,如`requestStore.ts`,并引入`createStore`和`usePersistedState`。
```typescript
import { createStore } from '@pinia/store';
import { usePersistedState } from 'vue3-persist-storage';
export const requestStore = createStore({
id: 'requestStore',
state: () => ({
loading: false,
data: null,
error: null,
}),
getters: {
// ...getters for your state
},
actions: {
fetchData({ commit }, payload) {
commit('SET_LOADING', true);
this.$axios.get(payload.url).then(response => {
commit('SET_DATA', response.data);
commit('SET_LOADING', false);
}).catch(error => {
commit('SET_ERROR', error.message);
commit('SET_LOADING', false);
});
},
},
mutations: {
SET_LOADING(state, loading) {
state.loading = loading;
},
SET_DATA(state, data) {
state.data = data;
},
SET_ERROR(state, error) {
state.error = error;
},
},
});
// 使用usePersistedState在store初始化时恢复数据
const persistedData = usePersistedState(requestStore.state, {
key: 'requestStore'
});
requestStore.state = persistedData;
```
3. 在组件中使用:
- 导入store并注入到组件的setup函数中,然后通过store的actions发起请求。
```typescript
import { onMounted, ref } from 'vue';
import { useRequestStore } from './requestStore';
export default {
setup() {
const loading = ref(false);
const requestAction = useRequestStore();
onMounted(() => {
requestAction.fetchData({ url: 'your-api-url' });
});
// 当需要更新状态时,直接操作store,例如:
onUnmounted(() => {
requestAction.setData(null); // 清理缓存数据
});
return {
loading,
// ...其他组件内的暴露属性和方法
};
}
};
```
阅读全文
相关推荐
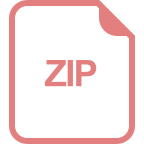
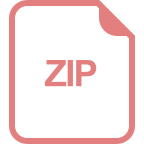
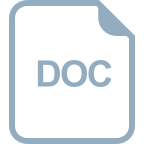

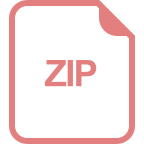
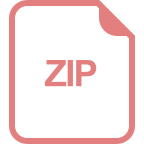
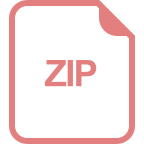
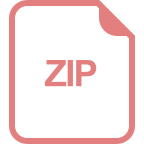
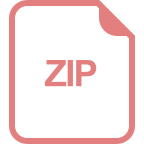
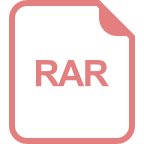
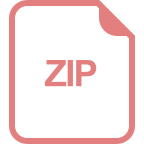
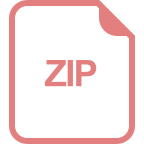
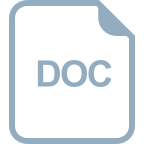



