c# 通过Shell脚本语言通信过程深入研究
时间: 2024-10-12 19:05:56 浏览: 32
在C#中,使用Shell脚本语言(如Windows的批处理bat或Unix/Linux的bash)进行通信通常涉及以下几个步骤:
1. **引入System.Diagnostics命名空间**:
C#标准库提供了一个`System.Diagnostics.Process`类,用于管理和控制外部进程。首先需要导入这个命名空间:
```csharp
using System.Diagnostics;
```
2. **创建Process对象**:
使用`Process.Start()`方法启动shell脚本,例如在Windows上启动一个.bat文件:
```csharp
string scriptPath = "path_to_your_script.bat";
ProcessStartInfo psi = new ProcessStartInfo(scriptPath);
psi.UseShellExecute = true; // 启动shell环境
Process process = new Process();
process.StartInfo = psi;
```
3. **异步执行或等待完成**:
可以通过`process.Start()`直接启动,或者设置`process.StartAsync()`进行异步执行。如果希望阻塞并等待脚本结束,可以用`WaitForExit()`方法:
```csharp
process.Start();
process.WaitForExit(); // 等待脚本执行完毕
```
4. **错误处理**:
脚本执行过程中可能会有错误,可以捕获异常信息:
```csharp
try
{
process.Start();
process.WaitForExit();
}
catch (Exception ex)
{
Console.WriteLine($"Script execution failed: {ex.Message}");
}
```
5. **传递参数**:
如果脚本需要接收参数,可以在`ProcessStartInfo.Arguments`中设置:
```csharp
psi.Arguments = "arg1 arg2"; // 添加命令行参数
```
6. **读取输出**:
你可以通过`StandardOutput.ReadToEnd()`或监听`Process.OutputDataReceived`事件来获取脚本的输出结果。
注意:在跨平台开发时,如果目标是Linux或Mac,你需要使用`.sh`脚本,并使用`Process`类的相应属性调整。
阅读全文
相关推荐
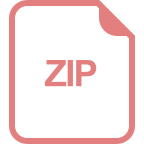
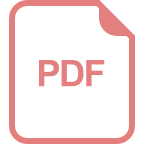
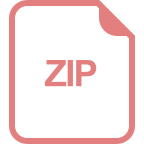
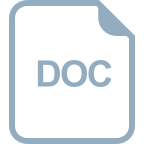
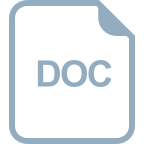
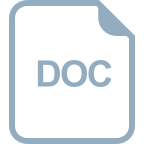
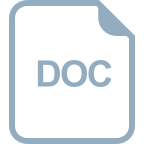
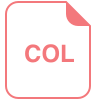
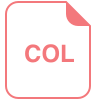
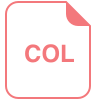
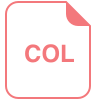
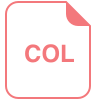
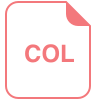
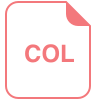
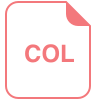
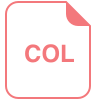
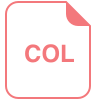
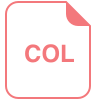
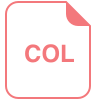