用C语言写个密码管理器,用数据结构存储用户的密码信息和账号信息。实现密码的生成、存储、查询和加密等功能
时间: 2024-10-17 15:09:17 浏览: 40
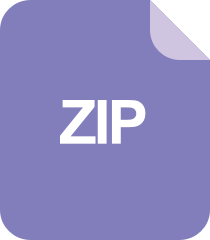
C语言控制台密码本项目
使用C语言编写密码管理器,你可以考虑使用哈希表(如链表或数组关联)来存储账户信息,包括用户名和加密后的密码。这里我们展示一个简单的示例,使用`struct`来表示用户信息,`bcrypt`库来进行密码加密。注意,C标准库并不包含加密函数,所以我们需要引入外部库(例如`bcrypt-c`)。
首先,我们需要安装`bcrypt-c`库并链接到项目中:
```sh
git clone https://github.com/dchest/bcrypt-c.git
cd bcrypt-c
make
sudo make install
```
然后,在C文件中使用`bcrypt_pbkdf()`函数:
```c
#include <stdio.h>
#include "bcrypt.h"
// 用户账户信息结构
typedef struct Account {
char username[50];
char hashed_password[BCRYPT_HASH_LEN];
char notes[100]; // 可选的额外信息
} Account;
// 加密密码
void encrypt_password(char *password, char *hashed_password) {
unsigned char salt[BCRYPT_SALT_LEN];
bcrypt_pbkdf(password, strlen(password), salt, BCRYPT_DEFAULT_COST, BCRYPT_HASH_LEN, hashed_password);
}
// 存储用户信息
void store_account(Account *accounts, int *size, char *username, char *plain_password, char *notes) {
Account new_account;
strcpy(new_account.username, username);
encrypt_password(plain_password, new_account.hashed_password);
strcpy(new_account.notes, notes);
accounts[*size] = new_account;
(*size)++;
}
// 查询用户信息
bool find_account(const Account *accounts, int size, char *username, char *hashed_password) {
for (int i = 0; i < size; i++) {
if (strcmp(accounts[i].username, username) == 0 && strcmp(accounts[i].hashed_password, hashed_password) == 0) {
return true;
}
}
return false;
}
int main() {
int size = 0;
Account accounts[10]; // 假设最多保存10个账户
while (true) {
printf("\n1. Store account\n2. Query account\n3. Exit\n");
int choice;
scanf("%d", &choice);
switch (choice) {
case 1:
char username[50], plain_password[50], notes[100];
printf("Enter username: ");
fgets(username, sizeof(username), stdin);
username[strlen(username) - 1] = '\0';
printf("Enter plaintext password: ");
fgets(plain_password, sizeof(plain_password), stdin);
plain_password[strlen(plain_password) - 1] = '\0';
printf("Enter notes: ");
fgets(notes, sizeof(notes), stdin);
store_account(accounts, &size, username, plain_password, notes);
break;
case 2:
char query_username[50], query_hashed_password[BCRYPT_HASH_LEN];
printf("Enter username to search: ");
fgets(query_username, sizeof(query_username), stdin);
query_username[strlen(query_username) - 1] = '\0';
printf("Enter hashed password: ");
fgets(query_hashed_password, sizeof(query_hashed_password), stdin);
query_hashed_password[strlen(query_hashed_password) - 1] = '\0';
bool found = find_account(accounts, size, query_username, query_hashed_password);
if (found)
printf("Found account.\n");
else
printf("Account not found.\n");
break;
case 3:
return 0;
default:
printf("Invalid option.\n");
}
}
return 0;
}
```
请注意,这只是一个基础示例,实际应用中你需要考虑更多的边缘情况,比如输入验证、错误处理和安全性增强(如使用安全的哈希算法和盐值)。
阅读全文
相关推荐
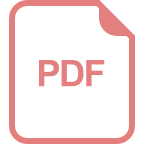
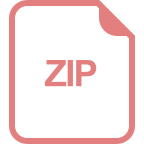
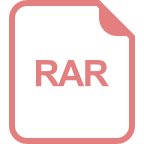
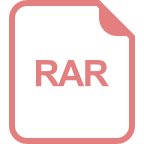
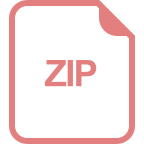
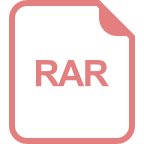
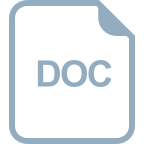
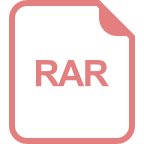
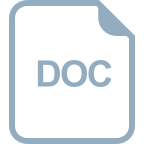
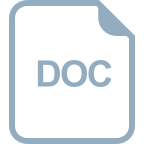
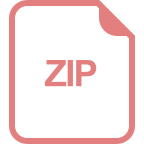
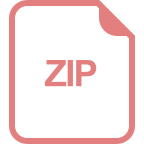