图片转换为16进制的c语言代码
时间: 2023-07-29 07:03:47 浏览: 390
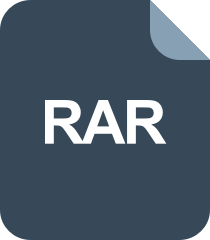
彩色图片转换16进制数据用此代码.rar_16进制 彩虹色_fpga图像处理

将图片转换为16进制的C语言代码可以通过以下步骤实现:
1. 首先,将图片文件读取到内存中。
2. 然后,逐个像素遍历图像,并将每个像素的RGB值转换为16进制。
3. 接下来,将16进制的颜色值存储在一个数组中。
4. 最后,将数组的内容输出为C语言代码的形式。
下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
FILE *fp;
unsigned char *image_data;
int width, height;
int i, j;
// 打开图片文件
fp = fopen("image.bmp", "rb");
if (fp == NULL) {
printf("无法打开图片文件!");
return 1;
}
// 获取图片的宽度和高度
fseek(fp, 18, SEEK_SET);
fread(&width, sizeof(int), 1, fp);
fread(&height, sizeof(int), 1, fp);
// 分配内存保存图片数据
image_data = (unsigned char *)malloc(width * height * 3);
// 读取图片数据
fseek(fp, 54, SEEK_SET);
fread(image_data, sizeof(unsigned char), width * height * 3, fp);
// 输出C语言代码
printf("unsigned char image_data[%d][%d][3] = {\n", height, width);
for (i = 0; i < height; i++) {
printf(" {\n");
for (j = 0; j < width; j++) {
printf(" {0x%02X, 0x%02X, 0x%02X},\n", image_data[(i * width + j) * 3], image_data[(i * width + j) * 3 + 1], image_data[(i * width + j) * 3 + 2]);
}
printf(" },\n");
}
printf("};\n");
// 释放内存并关闭文件
free(image_data);
fclose(fp);
return 0;
}
```
这段代码可以将名为`image.bmp`的图片文件转换为C语言代码,并以16进制的形式保存在`image_data`数组中。你可以根据需要自行修改文件名和输出格式。
阅读全文
相关推荐
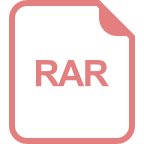
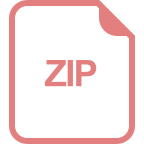
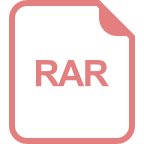
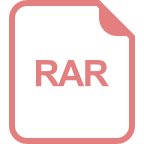
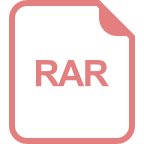
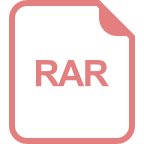
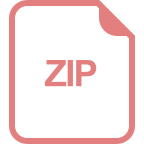
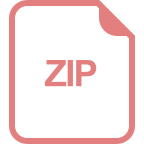
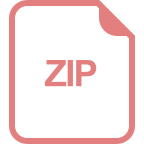
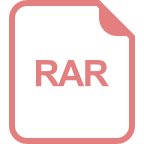
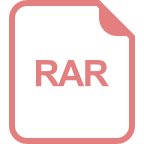
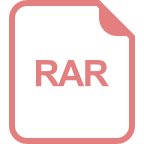
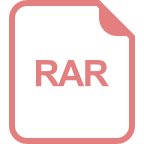
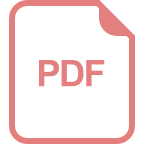
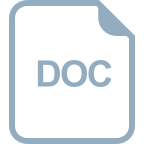