stream: true和长连接 的方式应该怎么写?
时间: 2024-04-18 11:26:19 浏览: 18
要使用 OpenAI API 的 stream 模式和长连接方式,您需要对代码进行一些修改。以下是使用 Java 进行 stream 请求和长连接的示例代码:
```java
import java.io.*;
import java.net.HttpURLConnection;
import java.net.URL;
public class OpenAIJavaStreamExample {
public static void main(String[] args) {
try {
String apiKey = "YOUR_API_KEY";
String prompt = "Hello, world!";
String model = "gpt-3.5-turbo";
OpenAIJavaStreamExample.sendRequest(apiKey, prompt, model);
} catch (IOException e) {
e.printStackTrace();
}
}
public static void sendRequest(String apiKey, String prompt, String model) throws IOException {
String url = "https://api.openai.com/v1/engines/" + model + "/completions";
URL obj = new URL(url);
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
// Set the request method and headers
con.setRequestMethod("POST");
con.setRequestProperty("Content-Type", "application/json");
con.setRequestProperty("Authorization", "Bearer " + apiKey);
// Enable streaming and set the initial prompt
con.setDoOutput(true);
OutputStreamWriter writer = new OutputStreamWriter(con.getOutputStream());
writer.write("{\"prompt\": \"" + prompt + "\", \"stream\": true}");
writer.flush();
// Read the response in a loop
BufferedReader reader = new BufferedReader(new InputStreamReader(con.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
System.out.println("Response: " + line);
// If you want to stop streaming at some point, you can break the loop based on certain conditions
if (line.contains("stop streaming condition")) {
break;
}
}
// Close the connections
writer.close();
reader.close();
con.disconnect();
}
}
```
请注意,与之前的代码相比,我们现在使用 `OutputStreamWriter` 来写入请求体,并使用 `BufferedReader` 来读取响应体。在读取响应的循环中,您可以根据需要添加逻辑来判断何时停止流式传输。
记得将 `YOUR_API_KEY` 替换为您自己的 API 密钥。
相关推荐
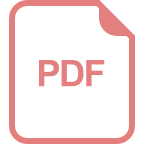
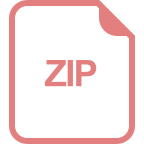
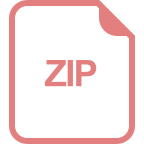














