输入身份证号,提取出生日期和年龄
时间: 2024-12-15 11:13:19 浏览: 7
在C语言中,直接操作身份证号码以提取出生日期并不是一个标准或推荐的做法,因为身份证号码的结构复杂,包含校验码等信息,通常不直接暴露敏感个人信息。但如果你确实需要进行这样的处理,一种常见的做法是在获取到身份证号码后,先验证其有效性(例如检查字符长度、校验码),然后根据已知的固定模式解析。
以下是一个简单的示例,使用字符串切片的方式来模拟这个过程,实际上这只是一个基础的演示,实际应用中应使用正则表达式库或者专门的身份证解析库:
```c
#include <stdio.h>
#include <string.h>
// 假设身份证号格式为:6位地区码 + 8位出生日期 + 4位顺序码 + 1位校验码
#define ID_DATE_LEN 8
void extract_id_info(const char *id) {
if (strlen(id) != 18 || id[17] != 'X' || !isdigit(id[0])) {
printf("Invalid ID number.\n");
return;
}
// 提取出生日期(年月日)
int birth_date[] = {atoi(id + 6), atoi(id + 7), atoi(id + 8)};
printf("Birth Date: %d-%d-%d\n", birth_date[0], birth_date[1], birth_date[2]);
// 提取当前年份并计算年龄
time_t now = time(0);
struct tm *t = localtime(&now);
int current_year = t->tm_year + 1900;
int age = current_year - birth_date[0];
if (current_year % 4 == 0 && (current_year % 100 != 0 || current_year % 400 == 0)) {
// 如果当前是闰年,考虑出生月份是否已经过完
if (t->tm_mon >= birth_date[1]) {
age--;
}
} else if (birth_date[1] > t->tm_mon || (birth_date[1] == t->tm_mon && birth_date[2] >= t->tm_mday)) {
// 非闰年,即使生日还没到也认为今年已经过了
age--;
}
printf("Estimated Age: %d years\n", age);
}
int main() {
const char *id_number = "110101199001015678"; // 示例身份证号
extract_id_info(id_number);
return 0;
}
```
注意:此代码仅用于演示目的,实际使用时应当确保符合当地法律法规对个人数据处理的规定。此外,这段代码没有处理所有可能的边界情况,比如出生日期的合法性检查等。
阅读全文
相关推荐
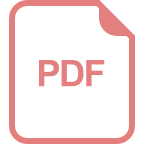
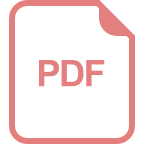
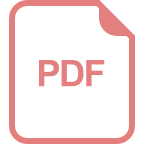
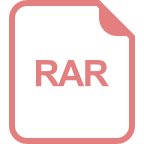
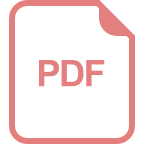
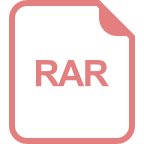
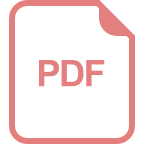
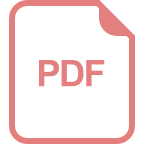
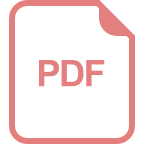
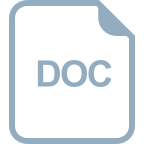
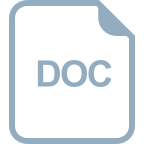







