为什么mybatisplus的service为空
时间: 2024-10-18 09:03:49 浏览: 45
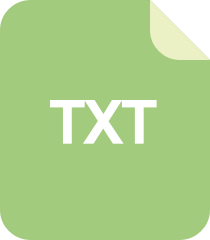
MybatisPlus
MyBatisPlus是一个基于MyBatis的简化ORM工具,它通常会自动生成Service和Repository等常用接口。如果遇到Service为空的情况,可能有以下几个原因:
1. **配置文件未启用**:确认是否在项目的全局配置文件中启用了MyBatisPlus的自动扫描功能,比如`BaseMapperScan`注解。
```java
@MapperScan("com.example.mapper") // 替换为你项目下的mapper包名
```
2. **生成的Service未注入**:检查是否有误将生成的Service组件注册到Spring容器中,例如在Spring Boot中可能需要添加`@ComponentScan`扫描相应路径。
3. **@Service注解缺失**:确保Service类上是否有`@Service`或其他Spring Bean注解,以便Spring能够识别并管理这个bean。
4. **构造函数注入或@Autowired未生效**:如果你手动创建了Service实例,确认是否通过构造函数注入了依赖,如果没有使用@Autowired注解的话,可能需要显式地设置依赖。
5. **包扫描范围问题**:如果在多模块项目中,可能是因为包扫描路径错误,没包括Service所在的包。
阅读全文
相关推荐
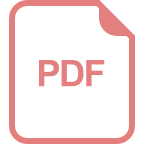
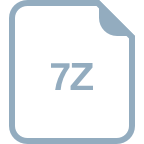
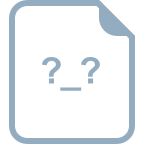
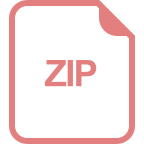
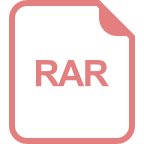












