回溯法0-1背包问题的最优解
时间: 2025-01-01 17:19:33 浏览: 27
### 使用回溯法求解0-1背包问题
#### 算法描述
对于给定的一组物品,每个物品有一个重量和价值,在限定总重量的前提下,如何选择这些物品使得所选物品的价值最大。使用回溯法解决此问题时,通过构建状态空间树来枚举所有可能的选择组合,并利用限界函数剪去不可能得到更优解的子树。
在最坏的情况下,计算上界`Bound()`函数需要O(n)时间,存在O(2^n)个右子节点需计算上界,因此解0-1背包问题的回溯算法`BackTrack`所需的计算时间为O(n*2^n)[^1]。
#### 实现代码示例
下面是一个简单的Python实现:
```python
def knapsack_backtrack(weights, values, capacity):
n = len(weights)
best_value = [0]
def backtrack(i, current_weight, current_value):
if i >= n or current_weight > capacity:
return
# Update the global optimal solution when reaching a leaf node.
if i == n - 1 and current_weight + weights[i] <= capacity:
if current_value + values[i] > best_value[0]:
best_value[0] = current_value + values[i]
if i == n - 1:
if current_value > best_value[0]:
best_value[0] = current_value
return
# Explore including this item
backtrack(i + 1, current_weight + weights[i], current_value + values[i])
# Or not include it (pruning based on bound can be added here)
backtrack(i + 1, current_weight, current_value)
backtrack(0, 0, 0)
return best_value[0]
weights = [2, 3, 4, 5]
values = [3, 4, 5, 6]
capacity = 8
print(f"The maximum value that can be put in a knapsack of capacity {capacity} is {knapsack_backtrack(weights, values, capacity)}")
```
上述程序定义了一个名为`knapsack_backtrack`的函数用于解决问题并打印出结果。该版本未加入具体的界限条件判断逻辑,实际应用中可以根据具体需求添加相应的优化措施以减少不必要的搜索路径。
阅读全文
相关推荐



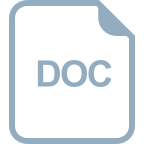

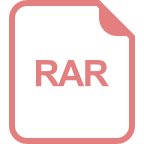










