帮我写一个记账的小程序,通过微信小程序原生开发软件实现
时间: 2024-02-27 13:55:03 浏览: 141
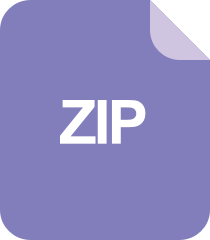
基于wepy开发的一款纯记账的微信小程序.zip
好的,开发一个记账小程序需要以下几个主要步骤:
1. 界面设计:使用微信小程序原生开发软件设计小程序的UI界面,包括首页、记账页面、报表页面等。
2. 数据库设计:设计小程序的数据表结构,包括账单表、分类表等。
3. 数据库操作:使用小程序提供的API进行数据库操作,包括增删改查等。
4. 记账功能实现:在记账页面中提供用户输入账单的功能,同时将账单存入数据库中。
5. 报表功能实现:在报表页面中对账单进行统计分析,并展示给用户。
下面是一个简单的代码示例:
1. 在app.js中定义全局变量和数据库初始化代码
```javascript
App({
globalData: {
userInfo: null,
db: null
},
onLaunch: function () {
// 初始化云数据库
wx.cloud.init({
env: 'xxx',
traceUser: true
})
// 获取数据库引用
const db = wx.cloud.database()
this.globalData.db = db
}
})
```
2. 在记账页面中实现账单的添加功能
```javascript
Page({
data: {
types: ['餐饮', '购物', '交通', '住房', '娱乐'],
typeIndex: 0,
amount: '',
remark: ''
},
bindTypeChange: function (e) {
this.setData({
typeIndex: e.detail.value
})
},
bindAmountInput: function (e) {
this.setData({
amount: e.detail.value
})
},
bindRemarkInput: function (e) {
this.setData({
remark: e.detail.value
})
},
addBill: function () {
const db = getApp().globalData.db
const { types, typeIndex, amount, remark } = this.data
const type = types[typeIndex]
if (!amount) {
wx.showToast({
title: '请输入金额',
icon: 'none'
})
return
}
db.collection('bill').add({
data: {
type,
amount: Number(amount),
remark,
time: new Date()
},
success: res => {
wx.showToast({
title: '记账成功'
})
this.setData({
typeIndex: 0,
amount: '',
remark: ''
})
},
fail: err => {
wx.showToast({
title: '记账失败',
icon: 'none'
})
console.error('[数据库] [新增记录] 失败:', err)
}
})
}
})
```
3. 在报表页面中实现账单的统计分析
```javascript
Page({
data: {
types: ['餐饮', '购物', '交通', '住房', '娱乐'],
typeIndex: 0,
totalAmount: 0,
bills: []
},
bindTypeChange: function (e) {
this.setData({
typeIndex: e.detail.value
})
this.getBillList()
},
getBillList: function () {
const db = getApp().globalData.db
const type = this.data.types[this.data.typeIndex]
db.collection('bill').where({
type
}).get({
success: res => {
const bills = res.data || []
const totalAmount = bills.reduce((sum, bill) => sum + bill.amount, 0)
this.setData({
bills,
totalAmount
})
},
fail: err => {
console.error('[数据库] [查询记录] 失败:', err)
}
})
},
onLoad: function () {
this.getBillList()
}
})
```
以上是一个简单的记账小程序的示例代码,您可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
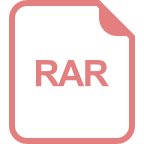
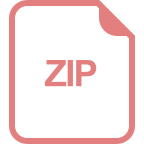
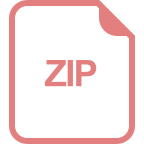
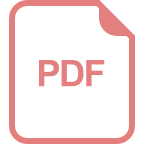
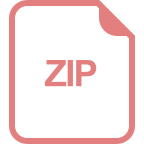
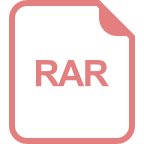
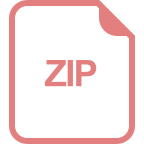
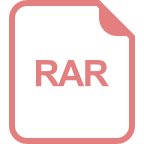
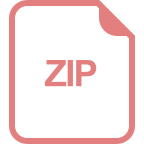
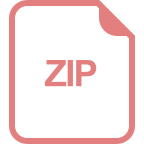
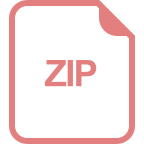
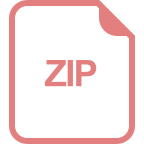
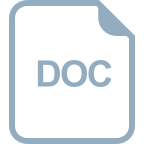
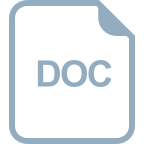
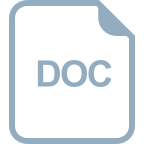
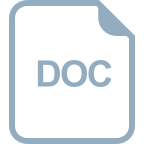