/*字符函数之求单词个数 定义单词为一串连续的字母.单词内不含非字母字符. 输入一个字符串,在函数内求串中单词的个数 输入输出样例: hello world total word= 2 程序的主要框架已经给出,请仅在 ********** Begin ********** ********** End *********** 注释对之间的空行中添加你的代码,任何修改该两行注释,以及修改注释外代码均将被判失败 */ #include <math.h> #include <stdio.h> #include <ctype.h> #define N 256 #define BUFSZ N int fun(char *p) { /********** Begin **********/ /********** End ***********/ } void f() { char s[256]; gets(s); printf("total word= %d\n",fun(s)); } int main() { f(); return 0; }
时间: 2023-07-05 22:27:30 浏览: 99
下面是代码实现:
```c
#include <stdio.h>
#include <ctype.h>
int fun(char *p) {
int count = 0, flag = 0;
while (*p) {
if (isalpha(*p)) { // 判断当前字符是否为字母
if (!flag) { // 如果当前字符是一个单词的开头,则单词数加一
flag = 1;
count++;
}
} else {
flag = 0; // 如果当前字符不是字母,则标记为单词结尾
}
p++;
}
return count;
}
void f() {
char s[256];
gets(s);
printf("total word= %d\n", fun(s));
}
int main() {
f();
return 0;
}
```
在上述代码中,我们使用了指针的方式来遍历字符串,用一个标志位flag来标记当前字符是否是一个单词的开头。如果当前字符是字母,则判断是否是单词开头,如果是,则单词数加一。如果当前字符不是字母,则标记为单词结尾。最后返回单词数。
希望能够帮助到你,如果还有其他问题,请随时提出。
阅读全文
相关推荐













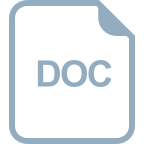

