c++中swap交换数组的用法
时间: 2023-05-27 07:01:49 浏览: 82
在C++中,可以使用一个名为std::swap的标准库函数来交换两个数组元素或变量的值。
语法如下:
```c++
#include <algorithm>
std::swap(a[index1], a[index2]);
```
其中,a是数组名,index1和index2是需要交换的两个元素的位置索引。
示例代码:
```c++
#include <iostream>
#include <algorithm>
using namespace std;
int main()
{
int a[5] = {1,2,3,4,5};
int index1 = 1;
int index2 = 3;
// 交换两个元素的值
swap(a[index1], a[index2]);
// 打印交换后的数组
for(int i=0; i<5; i++)
{
cout << a[i] << " ";
}
return 0;
}
```
输出结果:
```
1 4 3 2 5
```
在以上示例代码中,我们使用std::swap函数把索引1和3上的数组元素交换了位置。最终输出的数组中,原本在第2个位置上的数字2被交换到了第4个位置,原本在第4个位置上的数字4被交换到了第2个位置。代码执行结束后,a数组中的元素顺序就被修改了。
相关问题
linux c++ 如何释放vector数组的内存
释放 vector 数组的内存可以使用以下两种方法:
方法一:使用 vector 的 clear() 函数
可以使用 vector 的 clear() 函数来清空 vector 数组中的所有元素,释放内存。这个函数会调用每个元素的析构函数,从而释放元素所占的内存。示例代码如下:
```c++
std::vector<int> vec = {1, 2, 3, 4, 5};
vec.clear(); // 清空 vector 数组,释放内存
```
方法二:使用 vector 的 swap() 函数
另一种方法是使用 vector 的 swap() 函数,将 vector 与一个空的 vector 交换,从而释放 vector 所占的内存。示例代码如下:
```c++
std::vector<int>().swap(vec); // 释放 vector 数组的内存
```
这种方法会创建一个临时的空 vector,然后将其与原 vector 交换,从而释放原 vector 所占的内存。
c++ swap迭代器
c++中的swap操作可以用来交换两个相同类型容器的内容。例如,使用swap函数可以交换两个vector容器的内容。代码示例如下:
```
#include <vector>
#include <iostream>
int main() {
std::vector<std::string> vec1(10);
std::vector<std::string> vec2(24);
swap(vec1, vec2);
return 0;
}
```
上述代码中,我们创建了两个vector容器vec1和vec2,并分别初始化它们的大小为10和24。然后使用swap函数交换了它们的内容。这样,vec1中的内容变为原来的vec2的内容,vec2中的内容变为原来的vec1的内容。
此外,还有一种使用boost库中迭代器的swap操作的用法示例。代码示例如下:
```
#include <bits/stdc++.h>
#include "boost/iterator/iterator_adaptor.hpp"
using namespace std;
template<typename P>
class array_iter:public boost::iterator_adaptor<array_iter<P>,P> {
static_assert( is_pointer_v<P> ); //保证P是一个指针
public:
using super_type = typename array_iter::iterator_adaptor_;
array_iter(P x):super_type(x){} //必要的构造函数
};
int main() {
int a = {1, 2, 3};
array_iter<int*> start(a);
array_iter<int*> finish(a + 10);
start = 1;
copy(start, finish, ostream_iterator<int>(cout));
return 0;
}
```
上述代码中,我们使用了boost库中的iterator_adaptor来自定义了一个array_iter迭代器类。它是array_iter类的一个模板,并继承自iterator_adaptor。在main函数中,我们创建了一个int类型的数组a,并使用array_iter迭代器来遍历这个数组。在这个示例中,我们将数组中的每个元素都设置为1,然后使用copy函数将数组中的元素输出到标准输出流中。
因此,c++中的swap操作可以用于交换容器的内容,而迭代器可以用于遍历和访问容器的元素。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *3* [c++迭代器](https://blog.csdn.net/TOPEE362/article/details/126201656)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
- *2* [C++-swap](https://blog.csdn.net/mrbone11/article/details/121171745)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
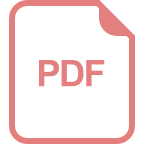












