c++ 获取当前进程所在目录
时间: 2023-05-02 09:06:32 浏览: 75
在Linux系统中,获取当前进程所在目录可以使用getcwd函数。该函数需要传入两个参数,第一个参数为一个字符数组,用于存放当前工作目录的路径,第二个参数为路径长度。代码如下:
#include <unistd.h>
int main() {
char path[1024];
if (getcwd(path, sizeof(path)) != NULL) {
printf("Current working directory: %s\n", path);
} else {
perror("getcwd() error");
}
return 0;
}
首先,定义一个长度为1024的字符数组path,用于存放当前进程所在目录的路径。然后,使用getcwd函数获取当前工作目录的绝对路径,并将其存储在path数组中。如果获取成功,则输出当前进程所在目录的路径;否则,打印出错信息。
相关问题
c++,获取当前进程所在目录
在 C++ 中,可以使用以下代码获取当前进程所在目录:
```c++
#include <iostream>
#include <windows.h>
int main() {
char buffer[MAX_PATH];
GetModuleFileName(NULL, buffer, MAX_PATH);
std::string::size_type pos = std::string(buffer).find_last_of("\\/");
std::cout << std::string(buffer).substr(0, pos) << std::endl;
return 0;
}
```
其中,`GetModuleFileName` 函数可以获取当前进程的可执行文件路径,`std::string::find_last_of` 函数可以查找字符串中最后一个出现指定字符的位置,`std::string::substr` 函数可以获取字符串的子串。最终输出的是该路径的目录部分。需要注意的是,这里使用了 Windows 平台下的 API 函数,如果在 Linux 平台下需要使用其他方式实现。
c++获取windows当前用户根目录
要获取Windows当前用户的根目录,可以使用下面的C++代码:
```cpp
#include <iostream>
#include <string>
#include <cstdlib>
std::string getHomeDirectory() {
char* homePath = getenv("USERPROFILE");
if (homePath == nullptr) {
homePath = getenv("HOMEDRIVE");
std::string homePathStr(homePath);
homePath = getenv("HOMEPATH");
homePathStr += homePath;
return homePathStr;
}
return std::string(homePath);
}
int main() {
std::string homeDirectory = getHomeDirectory();
std::cout << "Windows当前用户的根目录: " << homeDirectory << std::endl;
return 0;
}
```
这段代码使用了C++的getenv函数来获取环境变量USERPROFILE或HOMEDRIVE和HOMEPATH的值,它们都表示当前用户的根目录。如果USERPROFILE不可用,则使用HOMEDRIVE和HOMEPATH拼接而成。最后将获取到的根目录打印出来。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* [C++ 获取进程所在目录(全路径)](https://blog.csdn.net/lyshark_csdn/article/details/124938871)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *3* [c++ 获取当前用户的根目录](https://blog.csdn.net/u010953266/article/details/50340361)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
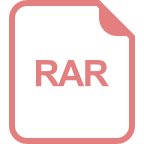
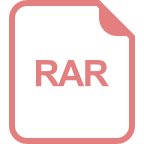








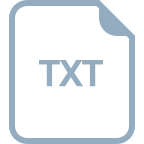
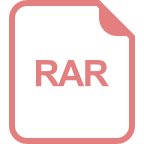
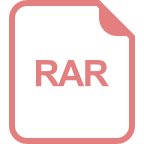
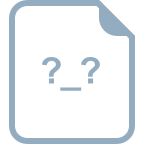
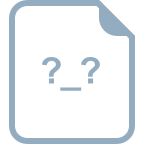