多文件下载压缩 客户端下载 java实例
时间: 2023-08-12 14:25:40 浏览: 100
以下是Java在客户端下载多个文件并压缩的示例代码:
```java
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
import javax.servlet.http.HttpServletResponse;
public class FileDownloader {
// 下载多个文件并压缩
public static void downloadFiles(List<String> fileNames, HttpServletResponse response) throws IOException {
// 设置响应头以便浏览器识别
response.setContentType("application/zip");
response.setHeader("Content-Disposition", "attachment; filename=files.zip");
// 创建ZipOutputStream对象
ZipOutputStream zipOut = new ZipOutputStream(response.getOutputStream());
// 遍历所有要下载的文件名
for (String fileName : fileNames) {
// 创建ZipEntry对象
ZipEntry zipEntry = new ZipEntry(fileName);
// 将ZipEntry对象添加到ZipOutputStream中
zipOut.putNextEntry(zipEntry);
// 创建输入流读取文件
BufferedInputStream bis = new BufferedInputStream(new FileInputStream(new File(fileName)));
// 创建缓冲区
byte[] buffer = new byte[1024];
int count = 0;
// 读取文件内容并写入ZipOutputStream
while ((count = bis.read(buffer)) != -1) {
zipOut.write(buffer, 0, count);
}
// 关闭输入流和ZipEntry对象
bis.close();
zipOut.closeEntry();
}
// 关闭ZipOutputStream对象
zipOut.close();
}
public static void main(String[] args) {
try {
// 创建要下载的文件名列表
List<String> fileNames = new ArrayList<>();
fileNames.add("file1.txt");
fileNames.add("file2.txt");
// 下载并压缩文件
downloadFiles(fileNames, response);
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在这个示例中,我们创建了一个名为`FileDownloader`的类,它包含一个名为`downloadFiles`的方法,该方法接受要下载的文件名列表和`HttpServletResponse`对象。该方法首先设置响应头以便浏览器识别,并创建`ZipOutputStream`对象。然后,它遍历所有要下载的文件名,为每个文件创建一个`ZipEntry`对象,并将其添加到`ZipOutputStream`中。接下来,它使用输入流读取文件内容,并将其写入`ZipOutputStream`中。最后,它关闭输入流、`ZipEntry`对象和`ZipOutputStream`对象,以便将压缩文件发送给客户端进行下载。
为了测试这个示例,我们可以在`main`方法中创建一些要下载的文件名,并调用`downloadFiles`方法来下载并压缩这些文件。请注意,`response`对象需要在调用`downloadFiles`方法之前进行初始化。
阅读全文
相关推荐
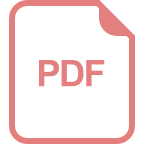
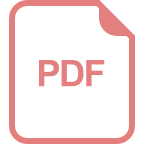
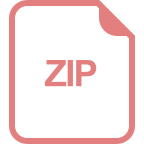















