用c语言写一个智能车冒泡排序
时间: 2024-03-08 14:44:05 浏览: 28
用C语言编写一个智能车冒泡排序的程序,可以实现对一组数字进行排序。冒泡排序是一种简单但效率较低的排序算法,它通过多次比较和交换相邻元素的方式将最大(或最小)的元素逐渐“冒泡”到数组的末尾。
下面是一个用C语言实现智能车冒泡排序的示例代码:
```c
#include <stdio.h>
// 定义智能车结构体
typedef struct {
int id;
int speed;
} SmartCar;
// 冒泡排序函数
void bubbleSort(SmartCar cars[], int n) {
int i, j;
for (i = 0; i < n-1; i++) {
for (j = 0; j < n-i-1; j++) {
if (cars[j].speed > cars[j+1].speed) {
// 交换相邻元素
SmartCar temp = cars[j];
cars[j] = cars[j+1];
cars[j+1] = temp;
}
}
}
}
int main() {
// 创建智能车数组
SmartCar cars[5] = {
{1, 50},
{2, 30},
{3, 70},
{4, 40},
{5, 60}
};
int n = sizeof(cars) / sizeof(cars[0]);
printf("排序前的智能车速度:\n");
for (int i = 0; i < n; i++) {
printf("智能车%d的速度:%d\n", cars[i].id, cars[i].speed);
}
// 调用冒泡排序函数
bubbleSort(cars, n);
printf("\n排序后的智能车速度:\n");
for (int i = 0; i < n; i++) {
printf("智能车%d的速度:%d\n", cars[i].id, cars[i].speed);
}
return 0;
}
```
这段代码中,我们首先定义了一个智能车的结构体,包含了智能车的ID和速度两个属性。然后,我们实现了一个冒泡排序函数`bubbleSort`,用于对智能车数组进行排序。在`main`函数中,我们创建了一个包含5辆智能车的数组,并调用`bubbleSort`函数对其进行排序。最后,我们输出排序前和排序后的智能车速度。
相关推荐
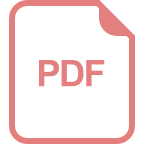
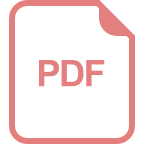









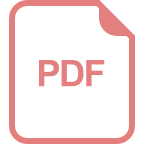
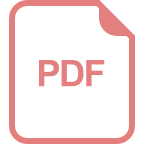
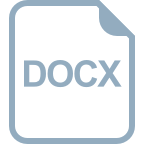