c#读写word文档
时间: 2023-07-27 22:18:54 浏览: 59
要在C#中读写Word文档,你可以使用Microsoft.Office.Interop.Word库,这是一个用于操作Word应用程序的COM接口。以下是一些基本的示例代码:
1. 打开Word应用程序
```csharp
using Microsoft.Office.Interop.Word;
Application wordApp = new Application();
```
2. 打开文档
```csharp
Document doc = wordApp.Documents.Open("文件路径");
```
3. 读取文档内容
```csharp
string text = doc.Content.Text;
```
4. 写入文档内容
```csharp
doc.Content.Text = "写入的文本内容";
```
5. 保存并关闭文档
```csharp
doc.Save();
doc.Close();
```
记得在使用完之后,释放Word应用程序资源。
```csharp
wordApp.Quit();
```
需要注意的是,使用Microsoft.Office.Interop.Word库需要安装Microsoft Office软件。
相关问题
c# 生成word文档并下载
你可以使用C#的Microsoft.Office.Interop.Word库来生成Word文档并进行下载。下面是一个简单的示例代码:
```csharp
using Microsoft.Office.Interop.Word;
// 创建Word文档
Application wordApp = new Application();
Document wordDoc = wordApp.Documents.Add();
// 添加内容到文档
Paragraph para = wordDoc.Paragraphs.Add();
para.Range.Text = "Hello, World!";
// 保存文档
string filePath = "path_to_save_document.docx";
wordDoc.SaveAs2(filePath);
// 关闭Word应用程序
wordDoc.Close();
wordApp.Quit();
// 下载文档
System.Web.HttpResponse response = System.Web.HttpContext.Current.Response;
response.ClearContent();
response.Clear();
response.ContentType = "application/msword";
response.AddHeader("Content-Disposition", $"attachment; filename={Path.GetFileName(filePath)}");
response.TransmitFile(filePath);
response.Flush();
response.End();
```
请确保你的项目引用了Microsoft.Office.Interop.Word库,并且拥有读写文件的权限。另外,这段代码中的下载部分是使用ASP.NET的方式进行下载,如果你是在其他类型的项目中使用,请根据相应的下载方式进行修改。
C# openxml读取word文档中指定书签标记的图表
可以使用C#的OpenXML SDK来读取Word文档中指定书签标记的图表。以下是大致的步骤:
1. 导入OpenXML SDK的命名空间。例如:`using DocumentFormat.OpenXml.Packaging;`和`using DocumentFormat.OpenXml.Wordprocessing;`。
2. 打开Word文档,并使用`WordprocessingDocument`类加载文档。例如:`WordprocessingDocument doc = WordprocessingDocument.Open("document.docx", true);`。
3. 获取文档中的书签标记。可以使用`BookmarkStart`和`BookmarkEnd`元素来识别书签标记。例如,使用以下代码获取名为“bookmark1”的书签标记:
```csharp
BookmarkStart bookmarkStart = doc.MainDocumentPart.Document.Body.Descendants<BookmarkStart>().Where(b => b.Name == "bookmark1").FirstOrDefault();
BookmarkEnd bookmarkEnd = doc.MainDocumentPart.Document.Body.Descendants<BookmarkEnd>().Where(b => b.Id.Value == bookmarkStart.Id.Value).FirstOrDefault();
```
4. 使用书签标记的位置信息,获取书签标记所在的段落和图表。例如,使用以下代码获取名为“bookmark1”的书签标记所在的段落和图表:
```csharp
Paragraph paragraph = bookmarkStart.Parent as Paragraph;
Drawing drawing = paragraph.Elements<Drawing>().FirstOrDefault();
ChartReference chartReference = drawing.Inline.Graphic.GraphicData.Descendants<ChartReference>().FirstOrDefault();
```
5. 获取图表数据,并使用相应的库(如OpenXML SDK、Excel Interop或EPPlus)进行分析和处理。例如,使用以下代码获取图表数据:
```csharp
ChartPart chartPart = (ChartPart)doc.MainDocumentPart.GetPartById(chartReference.Id);
OpenXmlReader reader = OpenXmlReader.Create(chartPart);
while (reader.Read())
{
if (reader.ElementType == typeof(SeriesText))
{
string seriesText = reader.GetText();
// 处理图表数据
}
}
```
请注意,这只是一个简单的示例,实际情况可能需要根据具体的文档和需求进行调整。
相关推荐
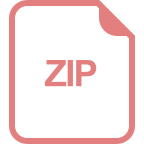
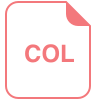
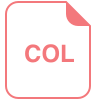
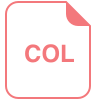
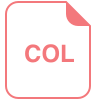
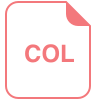







