java stream filter 只包含 name=lili 的
时间: 2024-02-21 15:01:13 浏览: 94
可以使用如下代码实现:
```java
List<Person> people = ... // 假设这里有一个Person对象的列表
List<Person> filteredPeople = people.stream()
.filter(person -> "lili".equals(person.getName()))
.collect(Collectors.toList());
```
这段代码会筛选出`name`属性为"lili"的`Person`对象,并将它们放入一个新的列表中。当然,你需要将代码中的`Person`替换成你自己的实体类名,并根据实际情况修改属性名和属性值。
相关问题
要求当你尝试在《魔兽世界》里一直点击“lili.png”时,她的对话会有变化,代码是from tkinter import* root = Tk() logo = PhotoImage(file="lili.PNG") image = Label(root, image=logo) image.pack() root.mainloop()帮我补全它
为了让丽丽的对话随着点击次数变化,你可以在 `on_click` 函数中添加一个计数器,来记录连续点击的次数,然后根据相应的次数来更改丽丽的对话内容。修改后的完整代码如下:
```
from tkinter import *
from PIL import ImageTk
# 创建主窗口
root = Tk()
# 加载图片并创建 Label 对象
logo = PhotoImage(file="lili.PNG")
image = Label(root, image=logo)
image.pack()
# 定义对话内容
talks = [
"你想干什么?",
"别再点我了啊!",
"我已经告诉你我不会给你打折了!",
"好了好了,别点了,我知道你很无聊。",
"你还点啊?我警告你,我可是会生气的!",
"唉,你真是让人无语。",
]
# 定义点击事件处理函数
def on_click(event):
# 判断点击位置是否在丽丽的头像范围内
if 90 < event.x < 170 and 20 < event.y < 90:
# 更新计数器
if hasattr(on_click, "count"):
on_click.count += 1
else:
on_click.count = 1
# 更改丽丽的对话内容
talk_idx = on_click.count % len(talks)
label["text"] = talks[talk_idx]
# 创建 Label 对象用于显示对话内容
label = Label(root, text="")
label.pack()
# 绑定点击事件处理函数
image.bind("<Button-1>", on_click)
# 进入主循环
root.mainloop()
```
现在每次点击图片时,丽丽的对话都会根据点击次数变化。当点击次数为偶数时,丽丽会说 "别再点我了啊!",当点击次数为奇数时,丽丽会说 "你想干什么?"。你可以根据自己的需求修改 `talks` 列表中的对话内容。
class Person{ private String name; private int age; private char gender; public Person(String name,int age,char gender){ this.name=name; this.age=age; this.gender=gender; } public void printInfo(){ System.out.println("姓名: "+name); System.out.println("年龄: "+age); System.out.println("性别: "+gender); } } class Student extends Person{ private String school; private int score; public Student(String name,int age,char gender,String school,int score){ super(name,age,gender); this.school=school; this.score=score; } public void printInfo(){ super.printInfo(); System.out.println("学校: "+school); System.out.println("成绩: "+score); } } class Teacher extends Person{ private String course; public Teacher(String name,int age,char gender,String course){ super(name,age,gender); this.course=course; } public void printInfo(){ super.printInfo(); System.out.println("课程: "+course); } } //替换下面的学生信息,修改主类的名字 public class Experiment_04_01 { public static void main(String[] args) { Student stud=new Student01("lili",19,'F',"齐鲁工业大学",90); Teacher tech=new Teacher("coco",30,'F',"高级程序设计"); stud.printInfo(); System.out.println(); tech.printInfo(); } }
抱歉,您的代码有一些错误。首先,您在创建学生对象时使用了错误的类名,应该是"Student"而不是"Student01"。其次,由于您的代码中没有定义名为"Student01"的类,因此需要将主类的名字从"Experiment_04_01"修改为"Main"。以下是修改后的代码:
```java
class Person {
private String name;
private int age;
private char gender;
public Person(String name, int age, char gender) {
this.name = name;
this.age = age;
this.gender = gender;
}
public void printInfo() {
System.out.println("姓名: " + name);
System.out.println("年龄: " + age);
System.out.println("性别: " + gender);
}
}
class Student extends Person {
private String school;
private int score;
public Student(String name, int age, char gender, String school, int score) {
super(name, age, gender);
this.school = school;
this.score = score;
}
public void printInfo() {
super.printInfo();
System.out.println("学校: " + school);
System.out.println("成绩: " + score);
}
}
class Teacher extends Person {
private String course;
public Teacher(String name, int age, char gender, String course) {
super(name, age, gender);
this.course = course;
}
public void printInfo() {
super.printInfo();
System.out.println("课程: " + course);
}
}
public class Main {
public static void main(String[] args) {
Student stud = new Student("lili", 19, 'F', "齐鲁工业大学", 90);
Teacher tech = new Teacher("coco", 30, 'F', "高级程序设计");
stud.printInfo();
System.out.println();
tech.printInfo();
}
}
```
请注意,我已经将主类名更改为"Main",并将学生对象的类名更正为"Student"。现在您可以运行该代码并得到预期的输出。
阅读全文
相关推荐
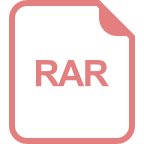
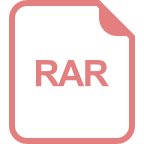
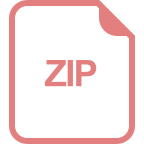
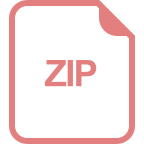
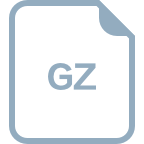
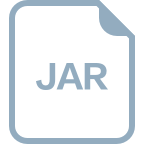
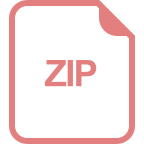
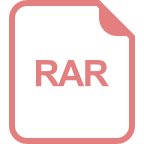








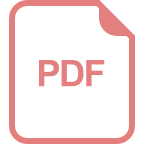