java怎么对比两个类的对象属性
时间: 2023-05-23 09:05:10 浏览: 309
A:Java可以通过实现Comparable接口或者使用Comparator来对比两个类的对象属性。
1. 实现Comparable接口
假设我们有一个Person类,它有两个属性:姓名和年龄。我们可以在Person类中实现Comparable接口,覆写compareTo方法,对Person对象进行比较。
```
public class Person implements Comparable<Person>{
private String name;
private int age;
public Person(String name, int age){
this.name = name;
this.age = age;
}
public int compareTo(Person p) {
return Integer.compare(this.age, p.getAge());
}
//Getter and Setter
}
```
在compareTo方法中,我们可以通过比较年龄属性的大小来判断两个Person对象的大小。如果返回正数,则表示当前对象大于参数对象;如果返回负数,则表示当前对象小于参数对象;如果返回0,则表示当前对象等于参数对象。
2. 使用Comparator
如果我们无法修改类的源代码,或者需要定制多种不同的比较规则,则可以使用Comparator接口。
假设我们仍然有一个Person类,我们可以新建一个PersonAgeComparator类来实现Comparator接口,覆写compare方法:
```
public class PersonAgeComparator implements Comparator<Person>{
public int compare(Person p1, Person p2) {
return Integer.compare(p1.getAge(), p2.getAge());
}
}
```
在compare方法中,我们同样比较两个Person对象的年龄属性,然后根据大小关系返回相应的值。
使用时,我们可以通过调用Collections.sort方法,将Person对象的List进行排序:
```
List<Person> persons = new ArrayList<>();
persons.add(new Person("Tom", 25));
persons.add(new Person("Lucy", 18));
Collections.sort(persons, new PersonAgeComparator());
```
上述代码将Person对象的List按照年龄升序排序。如果需要降序排序,只需将比较方法中返回值的正负号反转即可。
相关推荐
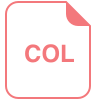
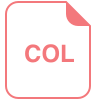
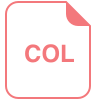
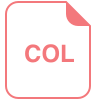
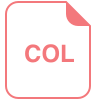









