用c++ 设计一个Bank类,实现银行某账户的资金往来账目管理。程序要求: 1)创建账户:包括账号、创建日期、余额 (创建账户时存入的钱数)。 2)存钱:执行存钱操作,并记录存钱日期和存钱数目。 3)取钱:执行取钱操作,并记录取钱日期和取钱数目。
时间: 2024-02-12 16:09:52 浏览: 83
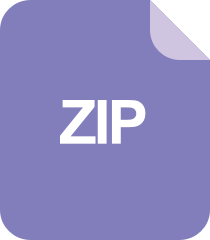
C++ 课程设计银行账户管理程序系统+源代码+文档说明+设计报告

以下是一个简单的Bank类的实现,可以满足你的需求:
```cpp
#include <iostream>
#include <string>
#include <ctime>
#include <vector>
class BankAccount {
public:
BankAccount(std::string account_number, double initial_balance) :
account_number_(account_number), balance_(initial_balance) {
time_t now = time(nullptr);
created_date_ = *localtime(&now);
}
void deposit(double amount) {
balance_ += amount;
time_t now = time(nullptr);
transaction_history_.push_back({ *localtime(&now), amount, "Deposit" });
}
void withdraw(double amount) {
if (amount > balance_) {
std::cout << "Not enough balance!" << std::endl;
return;
}
balance_ -= amount;
time_t now = time(nullptr);
transaction_history_.push_back({ *localtime(&now), amount, "Withdraw" });
}
void print_transaction_history() const {
for (const auto& transaction : transaction_history_) {
std::cout << asctime(&transaction.date) << " " << transaction.type << " " << transaction.amount << std::endl;
}
}
private:
struct Transaction {
tm date;
double amount;
std::string type;
};
std::string account_number_;
tm created_date_;
double balance_;
std::vector<Transaction> transaction_history_;
};
int main() {
BankAccount account("12345", 100.0);
account.deposit(50.0);
account.withdraw(30.0);
account.withdraw(200.0);
account.print_transaction_history();
return 0;
}
```
在BankAccount类中,我们定义了一个嵌套的Transaction结构体,用来记录每笔交易的日期、金额和类型(存款或取款)。在构造函数中,我们初始化了账户号码、创建日期和初始余额。deposit和withdraw方法分别用来执行存钱和取钱操作,并记录交易历史。print_transaction_history方法用来打印账户的交易历史。
在main函数中,我们创建了一个账户,执行了一些存钱和取钱操作,并打印了账户的交易历史。
阅读全文
相关推荐
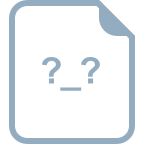
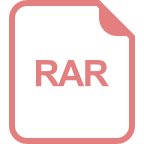
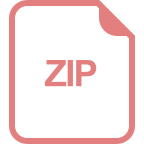
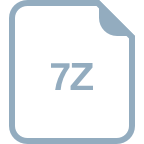
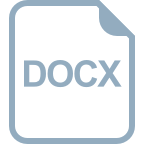
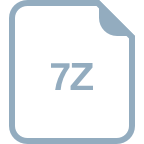
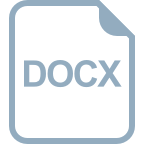