c++遍历文件夹中的图片
时间: 2023-07-16 10:16:42 浏览: 193
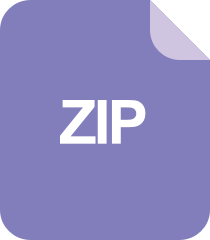
c++遍历文件夹
可以使用 C++ 的文件操作库 `dirent.h` 和图像处理库 `opencv` 来遍历文件夹中的图片。
首先,需要包含头文件:
```cpp
#include <dirent.h>
#include <opencv2/opencv.hpp>
```
然后,可以使用 `opendir` 和 `readdir` 函数遍历文件夹,使用 `cv::imread` 函数读取图片:
```cpp
DIR *dir;
struct dirent *ent;
if ((dir = opendir("your_folder_path")) != NULL) {
while ((ent = readdir(dir)) != NULL) {
std::string filename(ent->d_name);
if (filename.find(".jpg") != std::string::npos || filename.find(".png") != std::string::npos) {
// read image
cv::Mat image = cv::imread("your_folder_path/" + filename);
// do something with image
}
}
closedir(dir);
} else {
// handle error
}
```
注意,在读取图片时,需要判断文件名是否以 `.jpg` 或 `.png` 结尾。如果需要处理其他类型的图片,可以相应地修改判断条件。
完整代码示例:
```cpp
#include <iostream>
#include <dirent.h>
#include <opencv2/opencv.hpp>
int main() {
DIR *dir;
struct dirent *ent;
if ((dir = opendir("your_folder_path")) != NULL) {
while ((ent = readdir(dir)) != NULL) {
std::string filename(ent->d_name);
if (filename.find(".jpg") != std::string::npos || filename.find(".png") != std::string::npos) {
// read image
cv::Mat image = cv::imread("your_folder_path/" + filename);
if (image.empty()) {
std::cout << "Failed to read image " << filename << std::endl;
continue;
}
// do something with image
cv::imshow("Image", image);
cv::waitKey(0);
}
}
closedir(dir);
} else {
std::cout << "Failed to open directory" << std::endl;
return 1;
}
return 0;
}
```
阅读全文
相关推荐
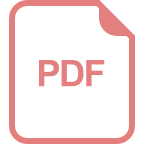
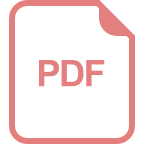













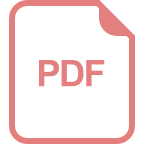