Java中httpmethod get=new getmethod(param)
时间: 2023-10-03 21:02:12 浏览: 111
在Java中,使用Apache HttpClient库可以发送http请求。其中,GET请求可以通过创建HttpGet实例来实现,具体代码如下:
```
HttpGet httpGet = new HttpGet(url + "?" + param); // 创建HttpGet实例
HttpResponse httpResponse = httpClient.execute(httpGet); // 发送http请求并接收响应
```
其中,url是请求的地址,param是请求所携带的参数。需要注意的是,param需要按照key1=value1&key2=value2的格式进行拼接。例如:
```
String url = "http://example.com/api";
String param = "id=123&name=Tom";
HttpGet httpGet = new HttpGet(url + "?" + param); // 创建HttpGet实例
HttpResponse httpResponse = httpClient.execute(httpGet); // 发送http请求并接收响应
```
这段代码会向http://example.com/api发送一个GET请求,请求参数为id=123&name=Tom。具体的http请求的响应可以通过HttpResponse对象获取。
相关问题
HttpMethod get =new Getmethod(param); get.getparams().setcontentcharset("UTF-8"); int statusCode; statusCode =httpclient.executeMethod(get); String bodyString =get.getResponseBodyAsString(); 上面是一段Java代码请按步骤详细解释并说明是否合理并给出理由
这段Java代码的功能是使用HttpClient库发送一个HTTP GET请求,并获取响应的HTTP状态码和响应体。
具体解释如下:
1. 第一行代码创建了一个HttpGet对象,该对象的构造方法需要传入一个URL参数,用于指定要发送请求的目标地址。
2. 第二行代码调用了HttpGet对象的getParams()方法,获取请求参数对象,然后使用setContentCharset()方法设置请求体的字符集为UTF-8。这一步是可选的,如果请求体中不包含中文或其他非ASCII字符,可以省略这一步。
3. 第三行代码创建了一个HttpClient对象,用于发送HTTP请求。
4. 第四行代码执行了HTTP GET请求,并将响应的HTTP状态码保存在statusCode变量中。
5. 第五行代码调用了HttpGet对象的getResponseBodyAsString()方法,获取响应体的字符串表示。
这段Java代码看起来是合理的,因为它使用了HttpClient库提供的标准API,而且按照常规的HTTP请求流程发送了一个GET请求并获取响应。唯一需要注意的是,如果请求的URL包含了特殊字符(比如空格、中文、特殊符号等),需要进行URL编码,否则可能会导致请求失败。
public class HttpComponentsClientRestfulHttpRequestFactory extends HttpComponentsClientHttpRequestFactory { @Override protected HttpUriRequest createHttpUriRequest(HttpMethod httpMethod, URI uri) { if (httpMethod == HttpMethod.GET) { return new HttpGetRequestWithEntity(uri); } return super.createHttpUriRequest(httpMethod, uri); } //核心代码 private static final class HttpGetRequestWithEntity extends HttpEntityEnclosingRequestBase { public HttpGetRequestWithEntity(final URI uri) { super.setURI(uri); } @Override public String getMethod() { return HttpMethod.GET.name(); } } } 复制代码 三、使用 复制代码 RestTemplate restTemplate = new RestTemplate(); //修改restTemplate的RequestFactory使其支持Get携带body参数 restTemplate.setRequestFactory(new HttpComponentsClientRestfulHttpRequestFactory()); HttpHeaders headers = new HttpHeaders(); headers.setContentType(MediaType.APPLICATION_JSON); Map map= new HashMap(); map.put("param", 123); HttpEntity<String> httpEntity = new HttpEntity<>(JSON.toJSONString(map), headers); ResponseEntity<String> exchange = restTemplate.exchange("your url", HttpMethod.GET, httpEntity, String.class);这样就可以啊
非常抱歉,我之前的回答有误。虽然HTTP协议规定了GET请求不应该带上body,但是HTTP客户端和服务器通常都会支持GET请求带上body的方式,因此RestTemplate也提供了这种支持。
以下是使用RestTemplate发起带有body的GET请求的示例代码:
```java
RestTemplate restTemplate = new RestTemplate();
String url = "http://example.com/api/getUser";
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON);
Map<String, String> params = new HashMap<>();
params.put("username", "test");
params.put("password", "123456");
HttpEntity<Map<String, String>> entity = new HttpEntity<>(params, headers);
ResponseEntity<String> response = restTemplate.exchange(url, HttpMethod.GET, entity, String.class);
String result = response.getBody();
```
在上面的代码中,我们首先创建了一个RestTemplate实例,并指定了请求的URL,然后创建了一个HttpHeaders对象,设置了Content-Type为application/json,接着创建了一个包含参数的Map对象,并将其作为参数创建了一个HttpEntity对象,最后使用exchange方法发起带有body的GET请求,并获取响应结果。
另外,如果你需要在RestTemplate中全局设置支持GET请求带有body,可以使用以下代码:
```java
RestTemplate restTemplate = new RestTemplate(new HttpComponentsClientHttpRequestFactory());
restTemplate.getMessageConverters().add(new MappingJackson2HttpMessageConverter());
restTemplate.getMessageConverters().add(new StringHttpMessageConverter());
```
其中,HttpComponentsClientHttpRequestFactory是支持GET请求带有body的HTTP请求工厂。
阅读全文
相关推荐
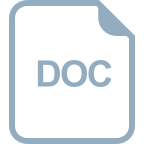
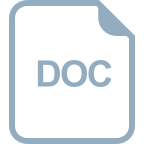
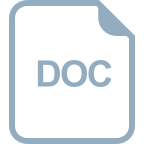
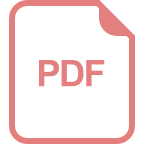
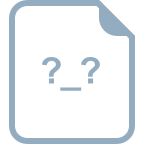
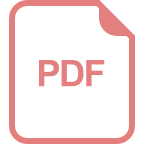
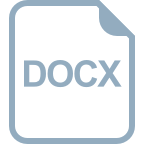
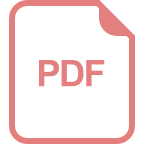
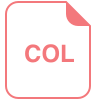
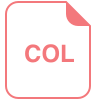
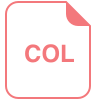





