文件上传的java代码
时间: 2023-10-12 14:20:44 浏览: 92
以下是文件上传的Java代码示例:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.UUID;
public class FileUploader {
public static void upload(String url, String filePath) throws IOException {
File file = new File(filePath);
String boundary = UUID.randomUUID().toString();
URL uploadUrl = new URL(url);
HttpURLConnection connection = (HttpURLConnection) uploadUrl.openConnection();
connection.setDoOutput(true);
connection.setDoInput(true);
connection.setRequestMethod("POST");
connection.setRequestProperty("Content-Type", "multipart/form-data; boundary=" + boundary);
String lineEnd = "\r\n";
String twoHyphens = "--";
// 开始写入文件
InputStream inputStream = new FileInputStream(file);
connection.getOutputStream().write((twoHyphens + boundary + lineEnd).getBytes());
connection.getOutputStream().write(("Content-Disposition: form-data; name=\"file\"; filename=\"" + file.getName() + "\"" + lineEnd).getBytes());
connection.getOutputStream().write(("Content-Type: application/octet-stream" + lineEnd + lineEnd).getBytes());
byte[] buffer = new byte[1024];
int length;
while ((length = inputStream.read(buffer)) != -1) {
connection.getOutputStream().write(buffer, 0, length);
}
connection.getOutputStream().write((lineEnd + twoHyphens + boundary + twoHyphens + lineEnd).getBytes());
inputStream.close();
// 获取响应信息
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
InputStream responseStream = connection.getInputStream();
byte[] responseBuffer = new byte[1024];
int responseLength;
while ((responseLength = responseStream.read(responseBuffer)) != -1) {
System.out.println(new String(responseBuffer, 0, responseLength));
}
responseStream.close();
} else {
System.out.println("上传失败,错误码:" + responseCode);
}
connection.disconnect();
}
}
```
使用示例:
```java
public static void main(String[] args) {
try {
FileUploader.upload("http://localhost:8080/upload", "/path/to/file");
} catch (IOException e) {
e.printStackTrace();
}
}
```
其中 `http://localhost:8080/upload` 是文件上传接口的 URL,`/path/to/file` 是文件路径。
阅读全文
相关推荐
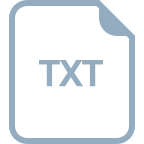
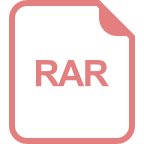
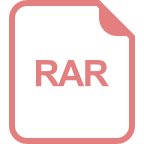
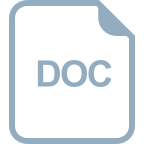
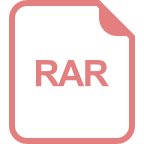
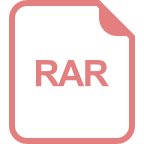
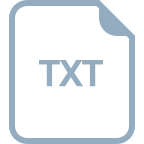
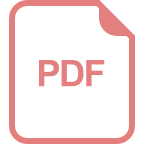
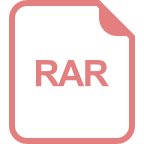
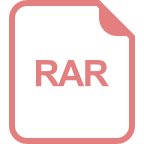