unity文件运行后如何移动视角
时间: 2024-10-10 09:09:21 浏览: 29
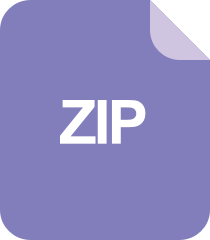
Unity UGUI第三人称视角手游 左侧控制移动,右侧控制视角和方向 (可根据自己需求修改)

在Unity中,移动游戏视角通常发生在第一人称或第三人称控制器场景中。以下是基本步骤:
1. **玩家控制**:如果你创建了一个第一人称角色,你可以通过`Input.GetAxis("Vertical")` 和 `Input.GetAxis("Horizontal")` 获取用户的键盘输入(例如WASD或方向键),然后更新角色的位置。例如:
```csharp
float moveSpeed = 5f; // 角色移动速度
Vector3 direction = new Vector3(Input.GetAxis("Horizontal"), 0, Input.GetAxis("Vertical"));
transform.position += direction * moveSpeed * Time.deltaTime;
```
2. **摄像机跟随**:对于固定视角或第三人称摄像机,你可以设置摄像机跟随角色。可以使用`transform.LookAt()` 或 `Camera.main.transform.position = player.transform.position` 来保持相机指向玩家。
3. **脚本绑定**:将上述代码添加到一个Update函数中,通常会关联到一个空物体或者摄像机上,以便于在每个帧都执行。
**相关问题--:**
1. 如何在Unity中创建并使用第一人称控制器?
2. Unity中有没有现成的组件可以直接控制摄像机跟随角色?
3. 如何限制摄像机的最大旋转角度?
阅读全文
相关推荐
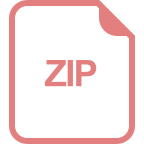
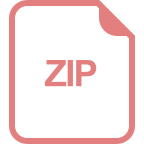
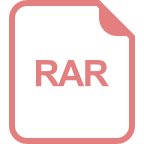
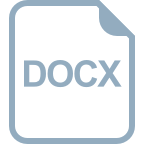
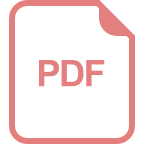
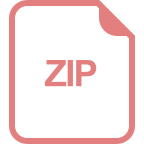
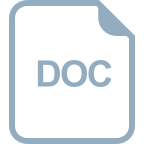
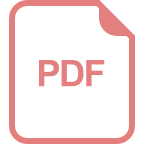
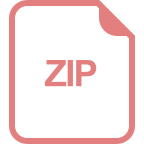
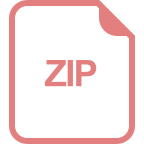
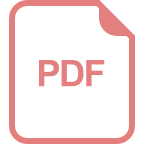
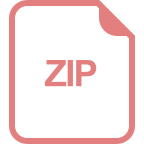
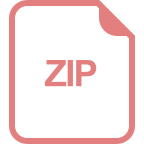
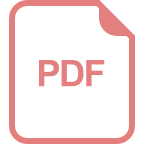
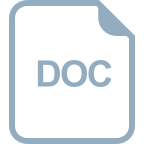
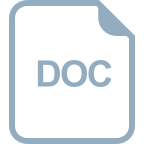
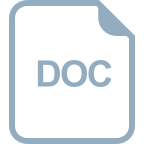
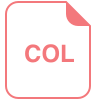