open_fifo_for_read windwos 替代
时间: 2023-11-23 08:07:20 浏览: 69
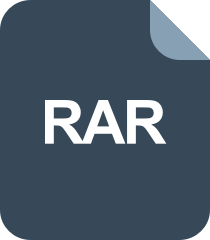
UNIX环境高级编程_第2版.part2

在 Windows 平台上,可以使用命名管道(Named Pipe)来替代 UNIX/Linux 上的 FIFO(Named Pipe 也可以在 UNIX/Linux 上使用)。Named Pipe 是一种有名的、双向的、命名的、匿名管道。与 FIFO 类似,Named Pipe 也可以用于进程间通信,但与 FIFO 不同的是,Named Pipe 可以在不同的进程之间进行双向通信。
以下是在 Windows 平台上使用 Named Pipe 创建一个读管道的示例代码:
```c++
#include <windows.h>
#include <stdio.h>
#define BUFFER_SIZE 1024
#define PIPE_NAME "\\\\.\\pipe\\testpipe"
int main() {
HANDLE hPipe;
char buffer[BUFFER_SIZE];
DWORD dwRead;
hPipe = CreateNamedPipeA(
PIPE_NAME,
PIPE_ACCESS_INBOUND,
PIPE_TYPE_MESSAGE | PIPE_READMODE_MESSAGE | PIPE_WAIT,
PIPE_UNLIMITED_INSTANCES,
BUFFER_SIZE,
BUFFER_SIZE,
0,
NULL
);
if (hPipe == INVALID_HANDLE_VALUE) {
printf("CreateNamedPipe failed with error %d\n", GetLastError());
return 1;
}
while (1) {
if (!ConnectNamedPipe(hPipe, NULL)) {
printf("ConnectNamedPipe failed with error %d\n", GetLastError());
CloseHandle(hPipe);
return 1;
}
if (ReadFile(hPipe, buffer, BUFFER_SIZE, &dwRead, NULL)) {
printf("Read %d bytes: %s\n", dwRead, buffer);
}
else {
printf("ReadFile failed with error %d\n", GetLastError());
}
DisconnectNamedPipe(hPipe);
}
CloseHandle(hPipe);
return 0;
}
```
该程序使用 CreateNamedPipeA 函数创建一个命名管道,并在管道上等待客户端连接。当客户端连接成功后,程序使用 ReadFile 函数从管道中读取数据,并输出到控制台。最后,程序使用 DisconnectNamedPipe 函数断开与客户端的连接。
你可以通过编写客户端程序来向该程序发送数据。方法与在 UNIX/Linux 上使用 FIFO 的方式相似,只需要使用 CreateFile、WriteFile、CloseHandle 等函数即可。
阅读全文
相关推荐
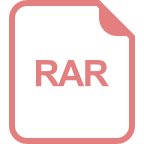
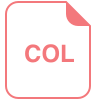
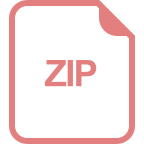
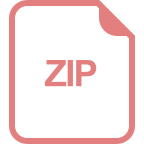
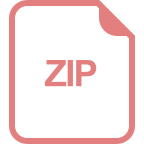
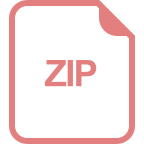
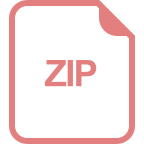
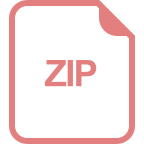
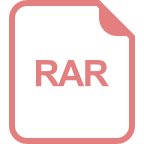
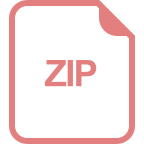
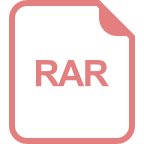
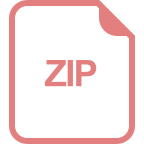