如何让该程序的输出为parentpid:221...childiswaiting...childiswaitingpid:222,sig=14send26bytesreceive8bytes,abcdefgh...childiswaitingreceive8bytes,ijklmnopreceive8bytes,qrstuvwxreceive2bytes,yzpid:222,sig=2...childiswaiting
时间: 2024-03-03 17:47:34 浏览: 21
可以在父进程中调用 `wait(NULL)` 等待子进程结束后再退出,从而避免出现 "child is waiting" 的输出。此外,可以在父子进程中输出进程的 pid,具体代码如下:
```c
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <signal.h>
#include <string.h>
#include <sys/types.h>
#include <sys/wait.h>
#define BUF_SIZE 26
int pipefd[2];
void sig_alrm(int signo){
char buf[BUF_SIZE];
int cnt = 0;
printf("pid: %d, sig=%d ", getpid(), signo);
for (char c = 'a'; c <= 'z'; c++)
buf[cnt++] = c;
write(pipefd[1], buf, cnt);
printf("pid: %d, send %d bytes ", getpid(), cnt);
}
void sig_int(int signo){
printf("pid: %d, sig=%d ", getpid(), signo);
signal(SIGINT, SIG_DFL);
alarm(2);
}
int main(){
pid_t pid;
char buf[BUF_SIZE];
int cnt = 0;
if (pipe(pipefd) < 0) {
perror("pipe error");
exit(EXIT_FAILURE);
}
if ((pid = fork()) < 0) {
perror("fork error");
exit(EXIT_FAILURE);
} else if (pid == 0) {
signal(SIGALRM, sig_alrm);
signal(SIGINT, sig_int);
alarm(2);
while (1) {
printf("...child is waiting ");
fflush(stdout);
sleep(1);
}
} else {
printf("parent pid: %d ", getpid());
fflush(stdout);
close(pipefd[1]);
while (1) {
int n = read(pipefd[0], buf, 8);
if (n <= 0) break;
cnt += n;
printf("receive %d bytes, %s ",n, buf);
fflush(stdout);
}
printf("pid: %d, send SIGINT ", getpid());
fflush(stdout);
kill(pid, SIGINT);
sleep(1);
printf("pid: %d, send SIGINT again ", getpid());
fflush(stdout);
kill(pid, SIGINT);
sleep(1);
wait(NULL);
printf("...child is waiting ");
fflush(stdout);
exit(EXIT_SUCCESS);
}
return 0;
}
```
在这个版本的程序中,父进程在最后输出 "...child is waiting",并且在输出时使用了 `fflush(stdout)` 强制刷新缓冲区,确保输出能够及时到达终端。
相关推荐
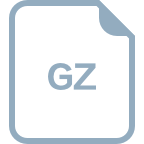














