请用c语言编写程序,输入年份和月份,输出该月的月历
时间: 2023-12-17 08:03:53 浏览: 86
以下是一个简单的 C 语言程序,用于输出指定年份和月份的月历:
```c
#include <stdio.h>
int is_leap_year(int year)
{
return ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0);
}
int get_days_in_month(int year, int month)
{
int days_in_month[] = {31, 28 + is_leap_year(year), 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
return days_in_month[month - 1];
}
void print_calendar(int year, int month)
{
int days_in_month = get_days_in_month(year, month);
int day_of_week = 1;
// Print the month and year
printf("%d/%d\n", month, year);
printf("Su Mo Tu We Th Fr Sa\n");
// Print the days of the month
for (int day = 1; day <= days_in_month; day++) {
// Print leading spaces for the first week
if (day == 1) {
for (int i = 1; i < day_of_week; i++) {
printf(" ");
}
}
// Print the day
printf("%2d ", day);
// Print a newline if it's the end of the week
if (day_of_week == 7 || day == days_in_month) {
printf("\n");
day_of_week = 1;
} else {
day_of_week++;
}
}
}
int main()
{
int year, month;
// Prompt the user for input
printf("Enter the year: ");
scanf("%d", &year);
printf("Enter the month: ");
scanf("%d", &month);
// Print the calendar
print_calendar(year, month);
return 0;
}
```
该程序使用 `is_leap_year` 函数判断是否为闰年,使用 `get_days_in_month` 函数获取指定月份的天数。接下来,程序使用 `print_calendar` 函数打印月历。在此函数中,程序首先打印月份和年份,然后打印每个日期。该函数使用一个计数器 `day_of_week` 跟踪每周的天数,并在每周的末尾打印一个换行符。
相关推荐
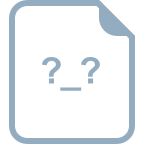














