用python生成一个可视化折半查找法
时间: 2024-09-23 13:15:07 浏览: 8
在Python中,我们可以使用matplotlib库来创建一个可视化折半查找法(Binary Search Algorithm)的流程图。首先,你需要安装`matplotlib`库,如果还没有安装,可以使用`pip install matplotlib`命令。
下面是一个简单的例子,展示如何用Python代码和matplotlib创建一个二分查找算法的动画:
```python
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
# 定义二分查找函数
def binary_search(array, target):
low = 0
high = len(array) - 1
while low <= high:
mid = (low + high) // 2
if array[mid] == target:
return mid
elif array[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1
# 创建一个示例数组
array = np.arange(0, 50)
# 初始化图像
fig, ax = plt.subplots()
ax.set_xlim([0, len(array)])
line, = ax.plot([], [], 'o', ms=10)
text = ax.text(0.02, 0.95, '', transform=ax.transAxes)
# 动画函数
def animate(i):
index = binary_search(array, i)
line.set_data(np.array([0, index]), np.array([i, i]))
text.set_text(f"Index: {index}, Target: {i}")
return line, text
# 创建动画并显示
ani = FuncAnimation(fig, animate, frames=np.arange(len(array)), interval=50)
plt.show()
相关推荐
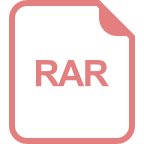
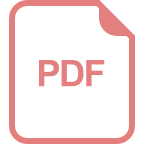
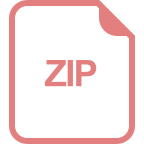














