conf_matrix = confusion_matrix(out, label, conf_matrix)
时间: 2024-06-13 15:06:43 浏览: 180
根据提供的引用内容,无法确定`confusion_matrix()`函数的具体实现。但是可以根据引用和引用中的代码推测,`confusion_matrix()`函数可能是一个用于计算混淆矩阵的函数。在引用中,`plot_confusion_matrix()`函数被调用,并传入了一个名为`cm_normalized`的参数,该参数可能是一个已经被归一化的混淆矩阵。在引用中,`plot_confusion_matrix()`函数被定义,并传入了一个名为`cm`的参数,该参数可能是一个未被归一化的混淆矩阵。因此,可以猜测`confusion_matrix()`函数可能是一个用于计算混淆矩阵的函数,该函数可能会返回一个混淆矩阵。
相关问题
def plot_confuse(model, x_val, y_val): predictions = model.predict_classes(x_val) truelabel = y_val.argmax(axis=-1) # 将one-hot转化为label conf_mat = confusion_matrix(y_true=truelabel, y_pred=predictions) plt.figure() plot_confusion_matrix(conf_mat, range(np.max(truelabel) + 1))
如果你正在使用Keras的Sequential模型,那么你需要使用predict()方法获取模型的预测结果,如下所示:
```python
import numpy as np
# 假设你的模型是一个Sequential对象,名为model
predictions = model.predict(x_val)
predicted_classes = np.argmax(predictions, axis=1)
truelabel = np.argmax(y_val, axis=1) # 将one-hot编码转化为标签形式
conf_mat = confusion_matrix(y_true=truelabel, y_pred=predicted_classes)
plt.figure()
plot_confusion_matrix(conf_mat, classes=range(np.max(truelabel) + 1))
```
这个代码将使用model的predict()方法获取模型的预测结果predictions,然后使用numpy.argmax()函数获取预测结果的类别predicted_classes。同时,使用numpy.argmax()函数将y_val从one-hot编码转化为标签形式,并保存在truelabel中。接下来,使用sklearn库中的confusion_matrix()函数计算混淆矩阵,并将其保存在conf_mat中。最后,使用自定义的plot_confusion_matrix()函数绘制混淆矩阵图。需要注意的是,classes参数应该是类别的列表,而不是类别标签的数组。
all_scores = []; n_samples = length(labels); n_class = length(unique(labels)); K = length(W); scores = cell(K,1); max_scores = zeros(K, n_samples); tmp_label = zeros(K, n_samples); for j=1:K projected_X = X * W{j}; scores{j} = projected_X * Y; [max_scores(j,:),tmp_label(j,:)] = max(scores{j}'); end [best_scores,best_idx] = max(max_scores,[],1); label_idx = sub2ind(size(tmp_label), best_idx, 1:n_samples); predict_label = tmp_label(label_idx); %compute the confusion matrix label_mat = sparse(labels,1:n_samples,1,n_class,n_samples); predict_mat = sparse(predict_label,1:n_samples,1,n_class, n_samples); conf_mat = label_mat * predict_mat'; conf_mat_diag = diag(conf_mat); n_per_class = sum(label_mat'); %mean class accuracy mean_class_accuracy = sum(conf_mat_diag ./ n_per_class') / n_class; %per sample accuracy mean_sample_accuracy = sum(conf_mat_diag) / n_samples;
这段代码是用于计算分类任务中的混淆矩阵和准确率的。让我解释一下它的逻辑:
首先,定义了一些变量,包括得分列表(all_scores)、样本数量(n_samples)和类别数量(n_class),以及一个矩阵W的长度(K)。
然后,创建了一些空的变量,包括得分矩阵(scores)、最大得分矩阵(max_scores)和临时标签矩阵(tmp_label)。
接下来,通过将输入矩阵X乘以每个权重矩阵W{j}来计算投影矩阵projected_X。然后,将投影矩阵projected_X与标签矩阵Y相乘,得到每个类别的得分矩阵scores{j}。
对于每个得分矩阵scores{j},找到每个样本的最大得分和对应的标签,并将它们存储在max_scores(j,:)和tmp_label(j,:)中。
接下来,找到所有样本中最大得分的索引和对应的标签,并将它们存储在best_scores和best_idx中。
使用best_idx和1:n_samples作为索引,从tmp_label中提取预测标签。
接下来,根据真实标签和预测标签创建稀疏矩阵label_mat和predict_mat,并计算它们的乘积,得到混淆矩阵conf_mat。
通过从混淆矩阵的对角线上获取元素,得到每个类别的正确预测数量conf_mat_diag。
计算每个类别的样本数量n_per_class。
计算平均类别准确率,即将每个类别的正确预测数量除以该类别的样本数量,并将结果相加后除以类别数量。
计算平均样本准确率,即将混淆矩阵对角线上的元素相加后除以样本数量。
这段代码的目的是评估分类模型的准确率和混淆矩阵,其中平均类别准确率是针对每个类别的样本数量进行加权的准确率指标,而平均样本准确率是针对所有样本的准确率指标。
阅读全文
相关推荐



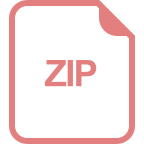
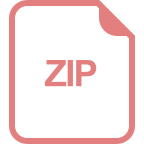
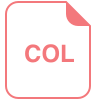




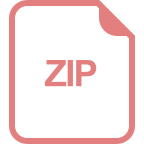
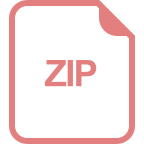
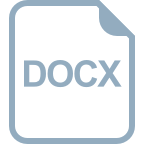
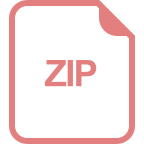