基于pytorch的Densene+bilstm+attention一维数据
时间: 2023-09-30 18:11:00 浏览: 192
如果你要使用PyTorch实现Dense-BiLSTM-Attention模型,可以按照以下步骤进行:
1. 加载数据:首先需要加载一维数据,并将其转换为PyTorch张量。你可以使用`torch.Tensor`或`torch.from_numpy`函数将数据转换为张量。
2. 定义模型:接下来,你需要定义一个包含Dense层、BiLSTM层和Attention层的模型。可以使用`nn.Module`类来定义模型,并在`__init__`函数中定义模型的各个层。可以使用`nn.Linear`定义Dense层,使用`nn.LSTM`定义BiLSTM层,使用`nn.Linear`定义Attention层。
3. 前向传播:在`forward`函数中实现模型的前向传播过程。首先通过Dense层将输入数据映射到一个高维空间,然后通过BiLSTM层对序列进行编码,最后通过Attention层计算每个时间步的权重,并将编码后的序列加权求和得到输出。
4. 训练模型:定义损失函数和优化器,并在训练集上训练模型。
5. 测试模型:使用测试集评估模型的性能。
下面是一个简单的示例代码,可以帮助你快速上手实现Dense-BiLSTM-Attention模型:
```python
import torch
import torch.nn as nn
# 加载数据
x = torch.randn(100, 1, 10) # 100个样本,1个通道,10个时间步
y = torch.randint(2, (100,))
# 定义模型
class DenseBiLSTMAttention(nn.Module):
def __init__(self, input_size, hidden_size, num_layers, num_classes):
super(DenseBiLSTMAttention, self).__init__()
self.dense = nn.Linear(input_size, hidden_size)
self.bilstm = nn.LSTM(hidden_size, hidden_size, num_layers, bidirectional=True)
self.attention = nn.Linear(hidden_size * 2, 1)
self.fc = nn.Linear(hidden_size * 2, num_classes)
def forward(self, x):
x = self.dense(x)
x, _ = self.bilstm(x)
x = x.view(-1, x.size(2))
alpha = torch.softmax(self.attention(x), dim=0)
x = (x * alpha).sum(dim=0)
x = self.fc(x)
return x
# 定义超参数
input_size = 10
hidden_size = 64
num_layers = 2
num_classes = 2
learning_rate = 0.001
# 定义模型、损失函数和优化器
model = DenseBiLSTMAttention(input_size, hidden_size, num_layers, num_classes)
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.Adam(model.parameters(), lr=learning_rate)
# 训练模型
num_epochs = 10
for epoch in range(num_epochs):
outputs = model(x)
loss = criterion(outputs, y)
optimizer.zero_grad()
loss.backward()
optimizer.step()
if (epoch+1) % 1 == 0:
print('Epoch [{}/{}], Loss: {:.4f}'.format(epoch+1, num_epochs, loss.item()))
# 测试模型
with torch.no_grad():
outputs = model(x)
_, predicted = torch.max(outputs.data, 1)
correct = (predicted == y).sum().item()
total = y.size(0)
print('Accuracy: {:.2f}%'.format(100 * correct / total))
```
这个代码实现了一个包含1个Dense层、2个BiLSTM层和1个Attention层的模型,可以用于分类任务。你可以根据自己的需求修改模型结构、超参数等。
阅读全文
相关推荐
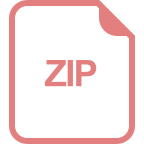
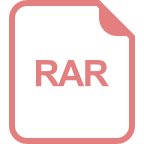
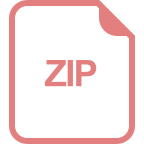
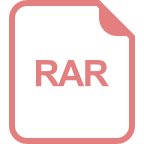
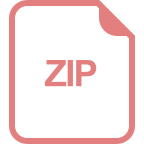
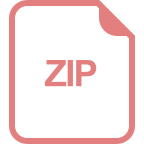
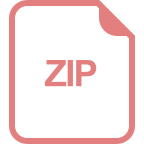
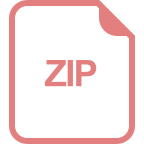
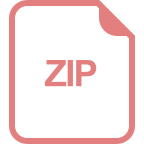
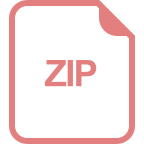
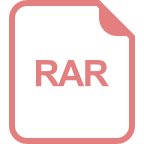
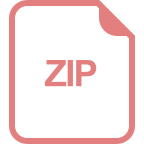
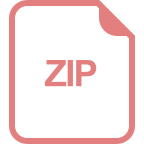
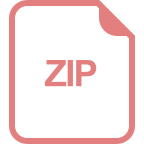
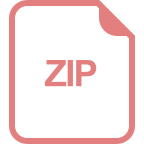
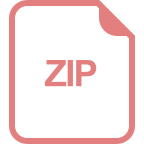
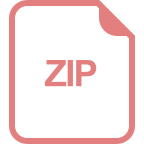