使用c语言实现
时间: 2023-10-03 15:09:14 浏览: 45
以下是使用C语言实现中缀表达式转后缀表达式的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_STACK_SIZE 100
typedef struct Stack {
char data[MAX_STACK_SIZE];
int top;
} Stack;
void init_stack(Stack *s) {
s->top = -1;
}
int is_empty(Stack *s) {
return s->top == -1;
}
int is_full(Stack *s) {
return s->top == MAX_STACK_SIZE - 1;
}
void push(Stack *s, char c) {
if (is_full(s)) {
printf("Stack Overflow!\n");
exit(1);
}
s->top++;
s->data[s->top] = c;
}
char pop(Stack *s) {
if (is_empty(s)) {
printf("Stack Underflow!\n");
exit(1);
}
char c = s->data[s->top];
s->top--;
return c;
}
char peek(Stack *s) {
if (is_empty(s)) {
printf("Stack Underflow!\n");
exit(1);
}
return s->data[s->top];
}
int is_operator(char c) {
return c == '+' || c == '-' || c == '*' || c == '/';
}
int precedence(char op) {
if (op == '*' || op == '/') {
return 2;
} else if (op == '+' || op == '-') {
return 1;
} else {
return 0;
}
}
void infix_to_postfix(char *infix_expr, char *postfix_expr) {
Stack op_stack;
init_stack(&op_stack);
int i = 0, j = 0;
while (infix_expr[i] != '\0') {
char c = infix_expr[i];
if (c >= '0' && c <= '9' || c >= 'a' && c <= 'z' || c >= 'A' && c <= 'Z') {
postfix_expr[j++] = c;
} else if (c == '(') {
push(&op_stack, c);
} else if (c == ')') {
while (peek(&op_stack) != '(') {
postfix_expr[j++] = pop(&op_stack);
}
pop(&op_stack);
} else if (is_operator(c)) {
while (!is_empty(&op_stack) && is_operator(peek(&op_stack)) && precedence(peek(&op_stack)) >= precedence(c)) {
postfix_expr[j++] = pop(&op_stack);
}
push(&op_stack, c);
}
i++;
}
while (!is_empty(&op_stack)) {
postfix_expr[j++] = pop(&op_stack);
}
postfix_expr[j] = '\0';
}
int main() {
char infix_expr[MAX_STACK_SIZE];
char postfix_expr[MAX_STACK_SIZE];
printf("Please enter an infix expression: ");
fgets(infix_expr, MAX_STACK_SIZE, stdin);
infix_expr[strlen(infix_expr) - 1] = '\0';
infix_to_postfix(infix_expr, postfix_expr);
printf("Postfix expression: %s\n", postfix_expr);
return 0;
}
```
示例输入:
```
Please enter an infix expression: A + B * C + D / E
```
示例输出:
```
Postfix expression: ABC*+DE/+
```
上述代码中,我们使用了一个结构体Stack来定义栈,并实现了栈的基本操作函数。在infix_to_postfix函数中,我们遍历中缀表达式的每一个字符,如果是操作数,则直接加入后缀表达式中;如果是左括号,则入栈;如果是右括号,则弹出栈顶运算符并加入后缀表达式中,直到遇到左括号为止;如果是运算符,则比较其与栈顶运算符的优先级,如果栈顶的优先级高,则弹出栈顶运算符并加入后缀表达式中,直到栈顶运算符的优先级低于当前运算符,最后将当前运算符入栈。最后,将栈中剩余的运算符全部弹出并加入后缀表达式中。
阅读全文
相关推荐
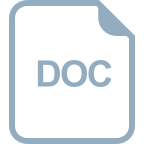
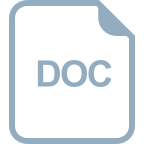
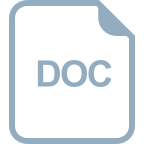