vue3+elementplus表单提交
时间: 2023-09-05 19:10:09 浏览: 66
首先,你需要使用Vue3和Element Plus来创建表单。你可以使用`el-form`和`el-form-item`来创建表单元素,同时使用`v-model`指令将表单元素与Vue组件的数据绑定起来。例如:
```html
<template>
<el-form :model="form" ref="form" :rules="rules" label-width="100px">
<el-form-item label="用户名" prop="name">
<el-input v-model="form.name"></el-input>
</el-form-item>
<el-form-item label="密码" prop="password">
<el-input type="password" v-model="form.password"></el-input>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="submitForm">提交</el-button>
</el-form-item>
</el-form>
</template>
<script>
import { ref } from 'vue';
import { ElForm, ElFormItem, ElInput, ElButton } from 'element-plus';
export default {
components: {
ElForm,
ElFormItem,
ElInput,
ElButton,
},
setup() {
const form = ref({
name: '',
password: '',
});
const rules = ref({
name: [{ required: true, message: '请输入用户名', trigger: 'blur' }],
password: [{ required: true, message: '请输入密码', trigger: 'blur' }],
});
const submitForm = () => {
// 表单提交逻辑
console.log('form submitted');
};
return {
form,
rules,
submitForm,
};
},
};
</script>
```
在这个例子中,我们使用了`ref`来创建`form`和`rules`变量,并将其与组件的模型和验证规则进行绑定。我们还定义了一个`submitForm`方法,在用户点击提交按钮时将会被调用。
在`submitForm`方法中,你可以使用`this.$refs.form.validate()`方法来验证表单是否合法,并在表单合法时执行表单提交逻辑。例如:
```javascript
const submitForm = () => {
this.$refs.form.validate((valid) => {
if (valid) {
// 表单提交逻辑
console.log('form submitted');
} else {
console.log('form not valid');
}
});
};
```
在这个例子中,我们使用了`validate`方法来验证表单是否合法,并在回调函数中判断表单是否合法。如果表单合法,我们就可以执行表单提交逻辑了。
希望这能对你有所帮助!
相关推荐
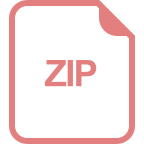
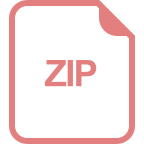
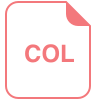
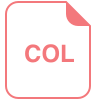
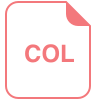
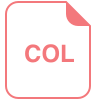
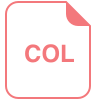









