node* CreateList(int array[], int n) { node *head = NULL, *tail = NULL; for (int i = 0; i < n; i++) { node* tmp = new node; tmp->data = array[i]; tmp->next = NULL; if (head == NULL) head = tail = tmp; else { tail->next = tmp; tail = tmp; } } return head; } 一句一句解释这段代码的作用
时间: 2024-04-10 08:31:56 浏览: 59
这段代码的作用是创建一个链表,并将给定的数组中的元素依次插入到链表中。
- `node* CreateList(int array[], int n)`:这是一个函数,接受一个整型数组 `array` 和数组长度 `n`,返回一个指向链表头部节点的指针。
- `node *head = NULL, *tail = NULL;`:定义了两个指针变量 `head` 和 `tail`,分别指向链表的头部和尾部。
- `for (int i = 0; i < n; i++)`:使用循环遍历数组中的元素。
- `node* tmp = new node;`:创建一个新的节点,并使用指针 `tmp` 指向该节点。
- `tmp->data = array[i];`:将当前数组元素的值赋给新节点的 `data` 域。
- `tmp->next = NULL;`:将新节点的 `next` 指针初始化为 `NULL`。
- `if (head == NULL)`:检查链表是否为空。如果链表为空,说明是第一个节点,将头部和尾部指针都指向新节点 `tmp`。
- `else`:如果链表不为空,则执行以下操作:
- `tail->next = tmp;`:将新节点 `tmp` 连接到尾部节点的下一个节点。
- `tail = tmp;`:更新尾部指针 `tail`,将其指向新的尾部节点 `tmp`。
- 循环结束后,返回头部指针 `head`,完成链表的创建和插入操作。
相关问题
#include <iostream> using namespace std; struct node { int data; node* next; }; node* createlist(int array[], int n); void printlist(node* head); int main() { int n; cin >> n; int array[100]; for (int i = 0; i < n; i++) { cin >> array[i]; } node* head = createlist(array, n); printlist(head); return 0; } node* createlist(int array[], int n) { node* head = NULL, * tail = NULL; for (int i = 0; i < n; i++) { node* tmp = new node; tmp->data = array[i]; tmp->next = NULL; if (head == NULL) head = tail = tmp; else { tail->next = tmp; tail = tmp; } } return head; } void printlist(node* head) { for (node* pi = head; pi != NULL; pi->next) { cout << pi->data << "-> "; } }该代码为何出现死循环
在函数`printlist`中,循环条件应该是`pi != NULL`,而不是`pi->next`。因为`pi->next`只是指向下一个节点,而没有将当前节点输出。因此,在遍历到最后一个节点时,`pi->next`为`NULL`,但是当前节点还没有被输出,因此会出现死循环。正确的循环条件应该是`pi != NULL`,即当当前节点为`NULL`时,退出循环。修改后的代码如下:
```
void printlist(node* head) {
for (node* pi = head; pi != NULL; pi = pi->next) {
cout << pi->data << "-> ";
}
}
```
优化#include <iostream> using namespace std; struct node { int data; node* next; }; node* createlist(int array[], int n) { node *head = NULL, * tail = NULL; for (int i = 0; i < n; i++) { node* tmp = new node; tmp->data = array[i]; tmp->next = NULL; if (head == NULL) head = tail = tmp; else { tail->next = tmp; tail = tmp; } } return head; } void printlist(node* head) { for (node* pi = head; pi != NULL; pi->next) { cout << pi->data << " "; } } int main() { int n; cin >> n; int array[100]; for (int i = 0; i < n; i++) { cin >> array[i]; } printlist(createlist(array, n)); return 0; }
在这段代码中,可以进行以下几个优化:
1. 头文件只需要包含 <iostream>,不需要使用整个 namespace std。
2. 在创建链表时,可以使用一个指针变量来代替 tail 指针,减少一个变量的使用。
3. 在打印链表时,循环条件应该是 pi != NULL,而不是 pi->next != NULL。
以下是优化后的代码:
```
#include <iostream>
using std::cin;
using std::cout;
struct node {
int data;
node* next;
};
node* createlist(int array[], int n) {
node *head = nullptr, *p = nullptr;
for (int i = 0; i < n; i++) {
node* tmp = new node;
tmp->data = array[i];
tmp->next = nullptr;
if (head == nullptr)
head = p = tmp;
else {
p->next = tmp;
p = tmp;
}
}
return head;
}
void printlist(node* head) {
for (node* pi = head; pi != nullptr; pi = pi->next) {
cout << pi->data << " ";
}
}
int main() {
int n;
cin >> n;
int array[100];
for (int i = 0; i < n; i++) {
cin >> array[i];
}
printlist(createlist(array, n));
return 0;
}
```
阅读全文
相关推荐
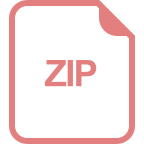
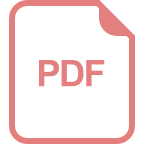
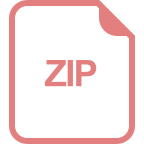
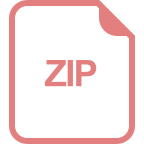
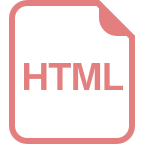
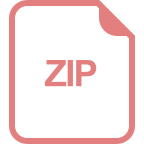
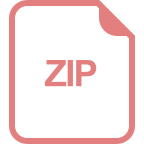
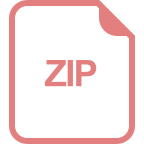
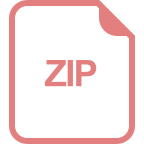
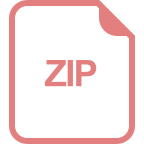
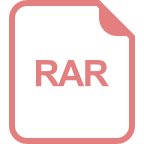
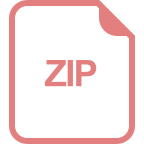
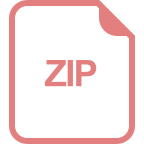
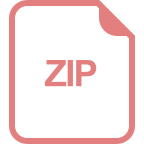