用opencv 的CUDA实现msr 图像增强
时间: 2024-05-10 17:18:30 浏览: 130
MSR (Multi-Scale Retinex) 图像增强是一种基于多尺度的图像增强算法,可以有效地改善图像的亮度、对比度和细节。而CUDA是一种利用GPU并行计算的技术,能够加速图像处理过程。
下面是使用OpenCV和CUDA实现MSR图像增强的步骤:
1. 导入必要的库
```python
import cv2
import numpy as np
from numba import cuda
```
2. 定义MSR算法函数
```python
@cuda.jit
def msr_cuda(img, output):
# 获取线程ID和块ID
x, y = cuda.grid(2)
tx, ty = cuda.threadIdx.x, cuda.threadIdx.y
bx, by = cuda.blockIdx.x, cuda.blockIdx.y
bw, bh = cuda.blockDim.x, cuda.blockDim.y
if x < img.shape[0] and y < img.shape[1]:
# 定义常数和参数
alpha = 125
beta = 46
sigma_list = [15, 80, 250]
eps = 1e-3
# 计算每个像素在不同尺度下的权重
for k in range(len(sigma_list)):
sigma = sigma_list[k]
weight = cuda.shared.array((3, 3), dtype=float32)
for i in range(3):
for j in range(3):
x_, y_ = x + i - 1, y + j - 1
if x_ >= 0 and x_ < img.shape[0] and y_ >= 0 and y_ < img.shape[1]:
weight[i, j] = 1.0 / (sigma * sigma) * np.exp(-((img[x_, y_] - img[x, y]) / alpha) ** 2 / 2)
else:
weight[i, j] = 0.0
weight /= (np.sum(weight) + eps)
# 计算在当前尺度下的像素值
value = 0
for i in range(3):
for j in range(3):
x_, y_ = x + i - 1, y + j - 1
if x_ >= 0 and x_ < img.shape[0] and y_ >= 0 and y_ < img.shape[1]:
value += weight[i, j] * np.log(img[x_, y_] + eps)
output[bx * bw + tx, by * bh + ty, k] = beta * np.exp(value)
```
3. 加载图像并将其转换为CUDA数组
```python
img = cv2.imread('test.jpg', cv2.IMREAD_GRAYSCALE)
img_cuda = cuda.to_device(img)
```
4. 定义Grid和Block大小
```python
threads_per_block = (32, 32)
blocks_per_grid_x = int(np.ceil(img.shape[0] / threads_per_block[0]))
blocks_per_grid_y = int(np.ceil(img.shape[1] / threads_per_block[1]))
blocks_per_grid = (blocks_per_grid_x, blocks_per_grid_y)
```
5. 创建输出数组并调用MSR函数
```python
output = cuda.device_array((img.shape[0], img.shape[1], 3))
msr_cuda[blocks_per_grid, threads_per_block](img_cuda, output)
```
6. 将CUDA数组转换为OpenCV图像并保存
```python
output_host = output.copy_to_host()
msr_img = np.zeros_like(img)
for k in range(3):
msr_img[:, :, k] = cv2.normalize(output_host[:, :, k], None, 0, 255, cv2.NORM_MINMAX, cv2.CV_8U)
cv2.imwrite('test_msr.jpg', msr_img)
```
完成上述步骤后,你就可以使用CUDA加速的MSR算法对图像进行增强了。
阅读全文
相关推荐

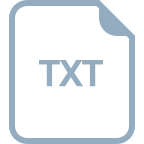
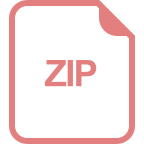
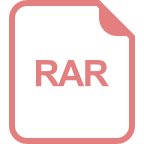
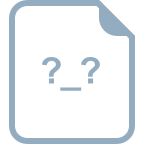
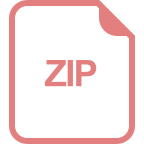
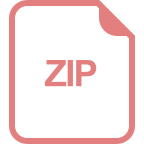
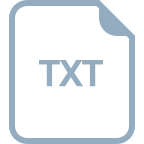
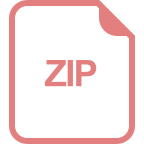
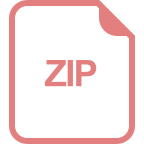
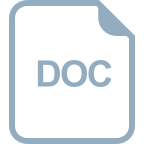


