字符串转为数字 C
时间: 2024-10-27 19:08:13 浏览: 67
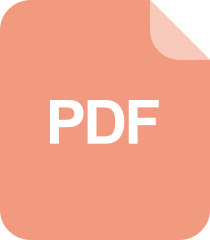
C语言实现将字符串转换为数字的方法
在C语言中,将字符串(通常是包含数字的字符串)转换为整数或浮点数的过程通常被称为“字符串解析”或“字符串到数字的转换”。这里有两个常见的方法:
1. **`atoi` 函数**:
使用C标准库中的`atoi`函数可以直接将包含整数的字符串转换为`int`类型的整数:
```c
#include <stdio.h>
int num = atoi("123"); // 将字符串 "123" 转换为整数 123
```
2. **自定义函数**:
如果`atoi`不可用或者需要更复杂的支持(比如浮点数),可以编写一个函数自行解析字符串,如利用`strtol`或`strtod`(针对长整型或双精度浮点数):
```c
#include <stdlib.h>
long long ll_num;
double db_num;
if (sscanf(str, "%lld", &ll_num) == 1) {
// 字符串str被解析为长整型
}
if (sscanf(str, "%lf", &db_num) == 1) {
// 字符串str被解析为双精度浮点数
}
```
这里`sscanf`函数用于逐个扫描并匹配输入的格式。
3. **错误处理**:
在处理用户输入或不确定来源的字符串时,一定要检查转换结果的有效性和范围,防止溢出或其他异常情况。
阅读全文
相关推荐
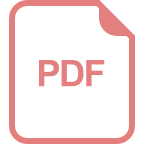
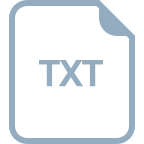








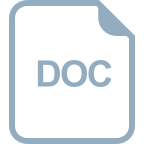






