用python语言写一个读取excel的第20,28列数据,将第20列数据作为x轴,第28列数据作为y轴,计算这些数据点的斜率,基于这些点的斜率大小进行分类,斜率最大的前百分之十作为一类,用红色点画出,斜率最小的百分之十作为一类,用绿色点画出,其余点用蓝色画出,可视化的代码
时间: 2024-03-20 12:42:10 浏览: 47
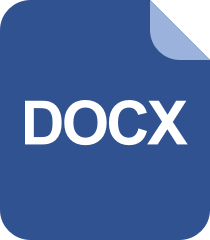
如何用python读取excel数据
好的,以下是实现你所需功能的Python代码:
```python
import openpyxl
import numpy as np
import matplotlib.pyplot as plt
# 读取excel数据
wb = openpyxl.load_workbook('data.xlsx')
sheet = wb.active
# 获取第20列和第28列的数据
x_values = []
y_values = []
for row in sheet.iter_rows(min_row=2, values_only=True):
x_values.append(row[19])
y_values.append(row[27])
# 计算斜率
slopes = []
for i in range(len(x_values)-1):
slope = (y_values[i+1] - y_values[i]) / (x_values[i+1] - x_values[i])
slopes.append(slope)
# 根据斜率分类
sorted_slopes = sorted(slopes)
num_of_points = len(sorted_slopes)
num_of_categories = 10
category_size = int(num_of_points / num_of_categories)
max_slopes = sorted_slopes[-category_size:]
min_slopes = sorted_slopes[:category_size]
# 可视化数据
fig, ax = plt.subplots()
for i in range(len(x_values)):
x = x_values[i]
y = y_values[i]
if slopes[i] in max_slopes:
ax.scatter(x, y, color='red')
elif slopes[i] in min_slopes:
ax.scatter(x, y, color='green')
else:
ax.scatter(x, y, color='blue')
plt.show()
```
需要注意的是,这里使用了openpyxl库来读取excel文件,因此需要先安装这个库。同时,这里默认excel文件中第1行是表头,数据从第2行开始。如果你的excel文件不符合这种格式,需要稍微修改一下代码。
阅读全文
相关推荐
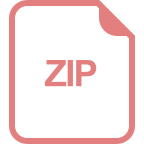
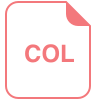
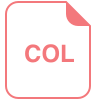
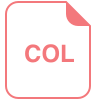
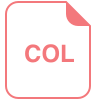
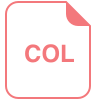
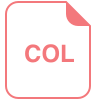
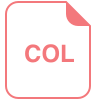
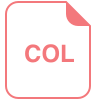
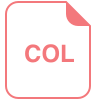
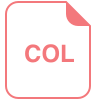
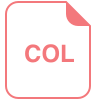
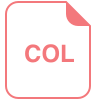
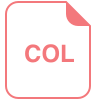
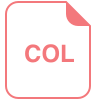
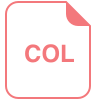
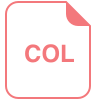