.net desktop runtime 和 .net framework区别
时间: 2024-01-07 08:04:08 浏览: 1330
.NET Desktop Runtime是.NET Core的一个组件,主要支持在Windows桌面应用程序上运行。.NET Framework是一个完整的框架,包含多个组件和库,支持在Windows桌面应用程序和ASP.NET Web应用程序上运行。.NET Desktop Runtime只是.NET Framework的一个子集,用于支持特定的Windows桌面应用程序。两者之间的差异主要在于功能的范围和目标平台的不同。
相关问题
.NET Framework 类库创建桌面快捷方式
要在Windows系统上利用.NET Framework创建桌面快捷方式,你可以使用C#的`System.Windows.Forms`命名空间,该命名空间包含了创建快捷方式所需的类。这里有一个简单的示例:
```csharp
using System;
using System.IO;
using System.Runtime.InteropServices;
using System.Windows.Forms;
namespace DesktopShortcutCreation
{
class Program
{
[DllImport("shell32.dll", SetLastError = true)]
static extern uint SHCreateShortcut(string pszFile, IntPtr pLink, string pszTargetPath, string pszArguments, string pszWorkingDirectory, uint fMask, IntPtr lpVerbArray, uint cVerb, IntPtr lpIconLocation, uint fFlags);
public static void Main()
{
// 文件路径和快捷方式名称
string desktopPath = Environment.GetFolderPath(Environment.SpecialFolder.Desktop);
string shortcutName = "MyProgram.lnk";
// 目标程序路径(如.exe文件)
string targetPath = @"C:\Path\To\Your\Executable.exe";
// 创建快捷方式
string shortcutFilePath = Path.Combine(desktopPath, shortcutName);
IntPtr link = Marshal.StringToHGlobalUni(targetPath);
uint result = SHCreateShortcut(shortcutFilePath, link, targetPath, "", "", 0, null, 0, IntPtr.Zero, 0x000F); // 创建文本链接
if (result != 0)
throw new Win32Exception(Marshal.GetLastWin32Error());
Console.WriteLine($"Desktop shortcut '{shortcutName}' created successfully.");
}
}
}
```
这段代码会创建一个指向指定应用程序的名为"MyProgram.lnk"的快捷方式,并将其放在桌面上。注意替换`targetPath`变量为你实际要创建快捷方式的目标程序路径。
.NET Framework 类库创建桌面图标快捷方式
在.NET Framework中,虽然`System.Windows.Forms.Design.Behavior`专注于Windows Forms组件的设计时配置,而不是直接创建桌面快捷方式,但是可以借助其他类库来实现这个需求。这里我们可以使用`System.IO`和`System.Diagnostics`来创建一个简单的快捷方式。
首先,我们需要明确一点,通常我们会在运行时而非设计时创建桌面快捷方式,因为这涉及到文件操作。以下是一个示例代码片段:
```csharp
using System;
using System.IO;
using System.Runtime.InteropServices; // 引入用于创建快捷方式的DllImport
public class DesktopShortcutCreator {
[DllImport("shell32.dll", SetLastError = true)]
private static extern bool ShellLinkCreateFromPath(string pszFile, out IntPtr psl);
private const string.desktopFolder = "C:\\Users\\%username%\\Desktop"; // 获取当前用户的桌面路径
public void CreateShortcut(string targetPath, string shortcutName) {
try {
// 创建ShellLink结构体
var lnkFileName = Path.Combine(desktopFolder, shortcutName + ".lnk");
IntPtr shellLink = IntPtr.Zero;
// 使用ShellLinkCreateFromPath创建快捷方式
if (ShellLinkCreateFromPath(targetPath, out shellLink)) {
using (var lnk = new SafeShellLinkObject(shellLink)) {
lnk.SetWorkingDirectory(Path.GetDirectoryName(targetPath)); // 设置工作目录
lnk.SetPath(targetPath); // 设置目标路径
lnk.Save(); // 保存快捷方式
}
} else {
Console.WriteLine($"Failed to create shortcut: {Marshal.GetLastWin32Error()}"); // 处理错误
}
Marshal.Release(shellLink); // 释放资源
} catch (Exception ex) {
Console.WriteLine($"Error creating shortcut: {ex.Message}");
}
}
}
```
要使用这个类,你可以这样实例化并调用它的方法:
```csharp
var desktopShortcut = new DesktopShortcutCreator();
desktopShortcut.CreateShortcut(@"C:\path\to\your\app.exe", "MyAppShortcut");
```
阅读全文
相关推荐
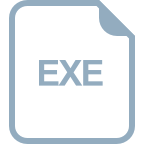
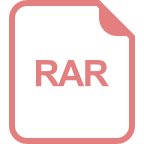
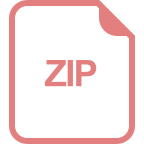
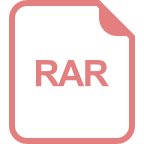
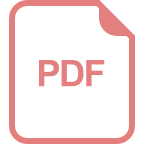
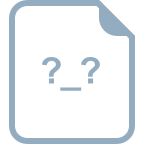
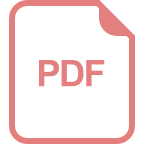
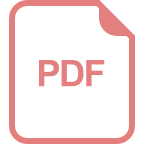
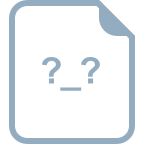
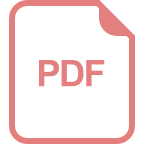
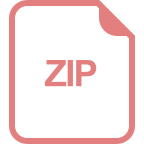
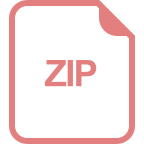
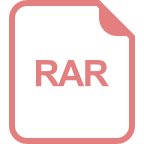
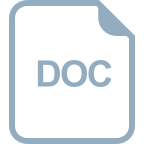

