请用c++实现:1.随机产生n(n>500)个整数,完成以下操作: (1)对给定的查找集合,顺序查找与给定值k相等的元素,并返回查找所用时间; (2)对给定的有序查找集合,折半查找与给定值k相等的元素,并返回查找所用时间;
时间: 2023-12-30 20:03:15 浏览: 67
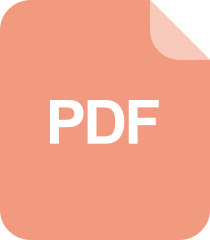
C语言/C++如何生成随机数

以下是用C++实现的代码:
```c++
#include <iostream>
#include <ctime>
#include <cstdlib>
#include <algorithm>
using namespace std;
// 顺序查找
double sequential_search(int a[], int n, int k) {
clock_t start, end;
start = clock();
for (int i = 0; i < n; i++) {
if (a[i] == k) {
end = clock();
return double(end - start) / CLOCKS_PER_SEC;
}
}
end = clock();
return double(end - start) / CLOCKS_PER_SEC;
}
// 折半查找
double binary_search(int a[], int n, int k) {
clock_t start, end;
start = clock();
int left = 0, right = n - 1;
while (left <= right) {
int mid = (left + right) / 2;
if (a[mid] == k) {
end = clock();
return double(end - start) / CLOCKS_PER_SEC;
} else if (a[mid] > k) {
right = mid - 1;
} else {
left = mid + 1;
}
}
end = clock();
return double(end - start) / CLOCKS_PER_SEC;
}
int main() {
const int n = 1000;
int a[n];
srand(time(NULL));
for (int i = 0; i < n; i++) {
a[i] = rand() % n;
}
int k = rand() % n;
// 顺序查找
double time1 = sequential_search(a, n, k);
cout << "Sequential Search Time: " << time1 << "s" << endl;
// 排序
sort(a, a + n);
// 折半查找
double time2 = binary_search(a, n, k);
cout << "Binary Search Time: " << time2 << "s" << endl;
return 0;
}
```
这个代码会生成一个包含 1000 个随机整数的数组,然后对该数组进行顺序查找和折半查找。你可以根据需要修改数组大小、搜索值等参数。
阅读全文
相关推荐
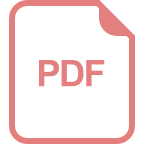



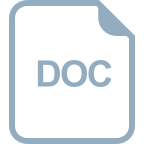
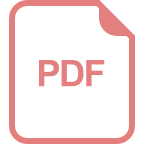
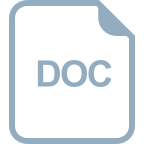
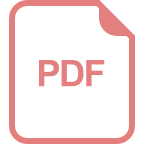
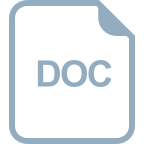
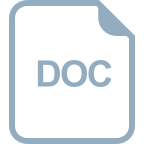
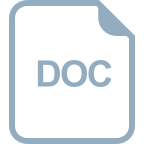
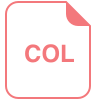
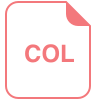
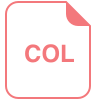
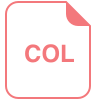
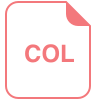
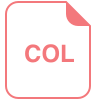