java RSA 公钥加密
时间: 2023-12-31 22:24:13 浏览: 141
在Java中使用RSA算法进行公钥加密的步骤如下:
1. 导入所需的类和包:
```java
import java.security.Key;
import java.security.KeyFactory;
import java.security.spec.X509EncodedKeySpec;
import javax.crypto.Cipher;
import java.util.Base64;
```
2. 定义RSA算法和密钥相关的常量:
```java
private final static String RSA_ALGORITHM = "RSA";
private final static String RSA_PUBLIC_KEY = "RSAPublicKey";
```
3. 获取公钥字符串,并将其转换为字节数组:
```java
String publicKeyStr = "这里填写你的公钥字符串";
byte[] publicKeyBytes = Base64.getDecoder().decode(publicKeyStr);
```
4. 根据公钥字节数组生成公钥对象:
```java
X509EncodedKeySpec keySpec = new X509EncodedKeySpec(publicKeyBytes);
KeyFactory keyFactory = KeyFactory.getInstance(RSA_ALGORITHM);
Key publicKey = keyFactory.generatePublic(keySpec);
```
5. 创建Cipher对象并进行加密操作:
```java
Cipher cipher = Cipher.getInstance(RSA_ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
byte[] encryptedBytes = cipher.doFinal(plainText.getBytes());
```
6. 将加密后的字节数组转换为Base64编码的字符串:
```java
String encryptedStr = Base64.getEncoder().encodeToString(encryptedBytes);
```
完整的示例代码如下:
```java
import java.security.Key;
import java.security.KeyFactory;
import java.security.spec.X509EncodedKeySpec;
import javax.crypto.Cipher;
import java.util.Base64;
public class RSAEncryptionExample {
private final static String RSA_ALGORITHM = "RSA";
private final static String RSA_PUBLIC_KEY = "RSAPublicKey";
public static void main(String[] args) throws Exception {
// 获取公钥字符串,并将其转换为字节数组
String publicKeyStr = "这里填写你的公钥字符串";
byte[] publicKeyBytes = Base64.getDecoder().decode(publicKeyStr);
// 根据公钥字节数组生成公钥对象
X509EncodedKeySpec keySpec = new X509EncodedKeySpec(publicKeyBytes);
KeyFactory keyFactory = KeyFactory.getInstance(RSA_ALGORITHM);
Key publicKey = keyFactory.generatePublic(keySpec);
// 加密操作
String plainText = "要加密的数据";
Cipher cipher = Cipher.getInstance(RSA_ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
byte[] encryptedBytes = cipher.doFinal(plainText.getBytes());
// 将加密后的字节数组转换为Base64编码的字符串
String encryptedStr = Base64.getEncoder().encodeToString(encryptedBytes);
System.out.println("加密后的数据:" + encryptedStr);
}
}
```
阅读全文
相关推荐






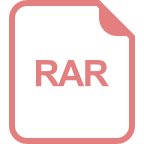











