request.AddParameter
时间: 2023-10-05 19:09:45 浏览: 56
request.AddParameter是一个方法,用于向HTTP请求中添加参数。在引用的代码示例中,通过这个方法向请求中添加了两个参数,分别是"format"和"param"。
在引用的代码示例中,使用了一个ParameterRequestWrapper类,该类用于对HttpServletRequest对象进行包装,以添加额外的参数。其中,addParameter方法用于向包装后的请求中添加参数。
在引用的代码示例中,创建了一个Parameters对象,该对象用于存储请求的参数。
可以看出,request.AddParameter的作用是向HTTP请求中添加参数,以便在发送请求时携带这些参数。
相关问题
var client = new RestClient("https://open.weixin.qq.com"); var request = new RestRequest("/connect/qrconnect", Method.GET); request.AddParameter("appid", appId); request.AddParameter("redirect_uri", redirectUri); request.AddParameter("response_type", "code"); request.AddParameter("scope", scope);这段代码翻译成java
以下是将上述 C# 代码翻译成 Java 的代码:
```java
import okhttp3.*;
import java.io.IOException;
public class Example {
public static void main(String[] args) throws IOException {
OkHttpClient client = new OkHttpClient();
HttpUrl.Builder urlBuilder = HttpUrl.parse("https://open.weixin.qq.com/connect/qrconnect").newBuilder();
urlBuilder.addQueryParameter("appid", appId);
urlBuilder.addQueryParameter("redirect_uri", redirectUri);
urlBuilder.addQueryParameter("response_type", "code");
urlBuilder.addQueryParameter("scope", scope);
String url = urlBuilder.build().toString();
Request request = new Request.Builder()
.url(url)
.build();
Response response = client.newCall(request).execute();
System.out.println(response.body().string());
}
}
```
上述 Java 代码使用了 OkHttp 库发送 HTTP 请求,并使用了 HttpUrl.Builder 对象构建请求的 URL,通过 addQueryParameter 方法添加请求参数。请求的 HTTP 方法为 GET,请求头和请求体均为空。发送请求并获取响应的过程也需要手动编写。
UNIGUI ajaxRequest
UNIGUI的ajaxRequest是用于在Web应用程序中进行异步请求的功能。它允许您通过JavaScript代码向服务器发送请求并接收响应,而无需刷新整个页面。
使用ajaxRequest,您可以向服务器发送数据,然后在收到响应后,通过回调函数处理返回的数据。这使得您能够在不中断用户界面的情况下更新特定部分的内容。
下面是一个示例代码,展示了如何使用ajaxRequest进行异步请求:
```delphi
procedure TForm1.Button1Click(Sender: TObject);
var
Request: TUniAjaxRequest;
begin
// 创建一个ajax请求
Request := TUniAjaxRequest.Create(Self);
// 设置请求的URL
Request.Url := 'http://example.com/api';
// 设置请求的方法(GET或POST)
Request.Method := amPost;
// 设置请求的参数
Request.AddParameter('param1', 'value1');
Request.AddParameter('param2', 'value2');
// 设置请求完成后的回调函数
Request.OnComplete :=
procedure(Response: TUniAjaxResponse)
begin
// 处理返回的数据
ShowMessage(Response.Content);
end;
// 发送请求
Request.Execute;
end;
```
在上面的示例中,我们创建了一个ajax请求,并设置了请求的URL、方法和参数。然后,我们定义了一个回调函数,在请求完成后处理返回的数据。最后,我们调用Execute方法发送请求。
请注意,上述示例仅为演示目的,并不代表完整的实现。具体使用方式可能会根据您的项目和需求有所不同。您可以根据UNIGUI的文档和示例代码进一步了解ajaxRequest的用法和功能。
相关推荐
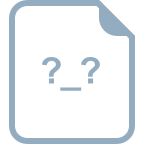
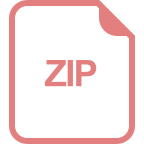












