perl 打印某数组
时间: 2024-10-11 18:04:21 浏览: 31
在Perl中,打印数组通常可以使用`print`函数配合数组元素的引用。这里是一个基本的例子,假设有一个名为`@my_array`的数组:
```perl
my @my_array = ('apple', 'banana', 'cherry');
# 使用foreach循环逐个打印数组元素
foreach my $item (@my_array) {
print "$item\n"; # 输出每个元素后换行
}
# 或者直接使用join方法连接元素并打印,省略换行符
print join(", ", @my_array), "\n"; # 输出:apple, banana, cherry
```
在这个例子中,`foreach`循环遍历数组中的每一个元素,并将其打印出来。如果你想要打印数组的所有元素而不需要换行,你可以使用`join`函数将它们连在一起,然后添加所需的分隔符,如逗号。
如果你想打印数组元素的某个范围,也可以这样做:
```perl
my @sub_array = @my_array[1..2]; # 获取从第二个到第三个元素的子数组
print "@sub_array\n"; # 输出:banana cherry
```
相关问题
perl中如果某元素不在数组中则执行操作
在Perl语言中,如果你想要检查某个元素是否存在于数组中,并在它不存在时执行特定的操作,你可以使用`exists`函数结合条件语句来实现。例如:
```perl
my @array = ('apple', 'banana', 'cherry');
if (! exists $array[0]) {
print "Element not found at index 0.\n";
# 执行其他操作...
} elsif ($array[0] eq 'apple') {
# 如果找到了,执行对应的处理...
}
# 或者使用数组下标查找并同时处理不存在的情况
if (my $value = $array{index}) {
# 如果找到值,执行...
} else {
print "Index $index is empty.\n";
}
```
在这个例子中,如果`$array[0]`不存在,程序会打印出相应的消息。如果元素存在并且等于预期的值,可以执行相关的操作。如果你尝试访问的是一个哈希而不是数组,记得要用`exists`而不是方括号[]。
生成perl语言某pattern 沿45度轴旋转功能的脚本
以下是一个示例 Perl 脚本,用于将给定图案沿 45 度轴旋转:
```perl
#!/usr/bin/perl
# Define the pattern to rotate
my @pattern = (
[0, 1, 0],
[1, 1, 1],
[0, 1, 0],
);
# Define the size of the pattern
my $size = scalar(@pattern);
# Create a new array to store the rotated pattern
my @rotated = ();
# Loop through each row and column of the pattern
for (my $row = 0; $row < $size; $row++) {
for (my $col = 0; $col < $size; $col++) {
# Calculate the new row and column indices for the rotated pattern
my $new_row = int(($row+$col)/2);
my $new_col = int(($col-$row+$size-1)/2);
# Add the rotated value to the new array
$rotated[$new_row][$new_col] = $pattern[$row][$col];
}
}
# Print the rotated pattern
for (my $row = 0; $row < $size; $row++) {
for (my $col = 0; $col < $size; $col++) {
print $rotated[$row][$col] ? 'X' : '.';
}
print "\n";
}
```
在这个示例中,我们定义了一个 3x3 的图案,然后使用两个嵌套的循环遍历每个元素。在内部循环中,我们计算了新的行和列索引,这些索引将旋转后的值添加到新的数组中。最后,我们打印出了旋转后的图案。
注意,在这个示例中,我们使用了整数除法运算符 `/` 来确保我们得到整数索引。如果你使用的是 Perl 5,你可能需要使用 `use integer` 来启用整数除法运算符。
阅读全文
相关推荐
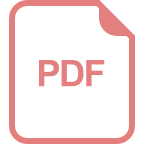
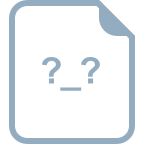
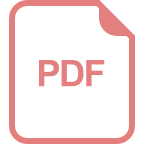
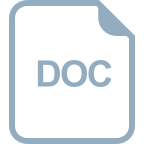
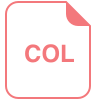
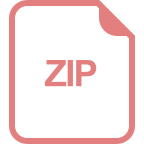
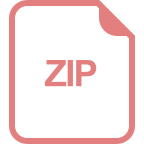
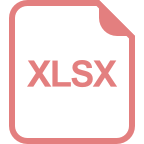
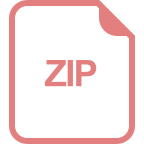
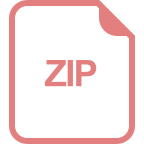
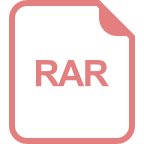
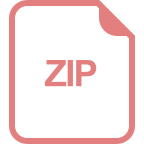