SM4 CBC 加密算法 PKCS5Padding c++ 实现
时间: 2024-12-01 13:19:45 浏览: 20
SM4 (Secure Hash Standard Message Algorithm 4) 是中国制定的一种对称加密算法,类似于AES,主要用于保护数据传输的安全。CBC (Cipher Block Chaining) 模式是一种常见的块密码模式,它通过将前一块的密文与当前明文块异或后再进行加密,增加了一定的保密性和安全性。
PKCS7Padding(也称为PKCS#5 Padding),是RSA Laboratories提出的填充标准,用于保证消息长度能被模钥位数整除。C++实现SM4 CBC加PKCS7Padding的过程需要库支持,如CryptoPP等,这里给出一个简化的伪代码概述:
```cpp
#include <openssl/aes.h>
#include <string>
std::string sm4_cbc_pkcs5_encrypt(const std::string& plaintext, const std::string& key, const std::string& iv) {
AES_KEY aesKey;
AES_set_encrypt_key(reinterpret_cast<const unsigned char*>(key.c_str()), 128, &aesKey); // SM4 key size is 128 bits
int paddingLength = key.length() % AES_BLOCK_SIZE; // PKCS5Padding length
char padding[AES_BLOCK_SIZE] = {0};
for (int i = 0; i < paddingLength; ++i)
padding[i] = static_cast<char>(paddingLength);
std::string paddedPlaintext(plaintext + std::string(padding, AES_BLOCK_SIZE));
unsigned char outputBuffer[AES_BLOCK_SIZE * (paddedPlaintext.size() / AES_BLOCK_SIZE + 1)];
memset(outputBuffer, 0, sizeof(outputBuffer)); // Initialize with zeros
AES_encrypt(reinterpret_cast<const unsigned char*>(paddedPlaintext.c_str()), outputBuffer, &aesKey, AES_ENCRYPT);
AES_encrypt(reinterpret_cast<const unsigned char*>(outputBuffer), outputBuffer, &aesKey, AES_NO_ENCRYPT); // Unencrypt the first block to apply IV
// Concatenate the ciphertext and IV for proper CBC chaining
return std::string(iv) + std::string(reinterpret_cast<char*>(outputBuffer), paddedPlaintext.size());
}
// 使用示例
std::string plainText = "Hello, World!";
std::string key = "MySecretKey123";
std::string iv = "RandomIV"; // SM4 CBC初始化向量
auto encrypted = sm4_cbc_pkcs5_encrypt(plainText, key, iv);
阅读全文
相关推荐
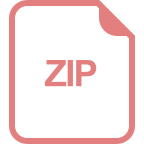
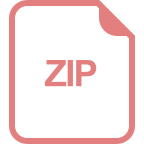
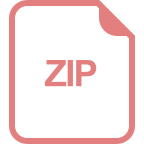

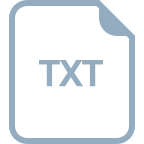
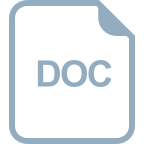












