文件中主要包含哪些springboot服务 怎么写
时间: 2024-12-17 08:29:45 浏览: 5
根据文档内容,《初设方案-20230815完善.docx》中并未详细列出具体的Spring Boot服务。不过,文档提到了一些与Spring Boot相关的技术和架构设计原则,这些可以为你编写Spring Boot服务提供指导。以下是文档中提到的关键点:
### 关键技术与架构设计原则
1. **微服务架构**:
- 文档采用了微服务架构风格,每个服务都是小型功能块,可以通过API集相互通信。
- 使用Spring Cloud、Dubbo或HSF作为微服务框架的基础支撑。
2. **Spring Cloud**:
- 利用Spring Boot的开发便利性简化分布式系统的开发。
- 使用Spring MVC提供的编程模型开发微服务。
- 使用Eureka实现服务注册和服务发现。
- 使用Feign调用微服务。
- 使用Hystrix实现限流熔断。
- 使用Zuul实现服务网关。
3. **Dubbo**:
- 高性能、轻量级的Java RPC框架。
- 提供面向接口的远程方法调用、智能容错和负载均衡、服务自动注册和发现。
- 支持zookeeper、nacos、edas实现服务注册和服务发现。
- 内置负载均衡、集群容错、熔断限流等能力。
4. **HSF**:
- 分布式服务框架,提供从分布式应用层面的支持。
- 使用ConfigServer或zookeeper实现服务注册和服务发现。
- 内置负载均衡、集群容错、限流熔断等能力。
### 示例Spring Boot服务
基于上述技术栈,你可以编写以下几个示例Spring Boot服务:
#### 1. 地名地址管理服务
```java
@SpringBootApplication
@EnableDiscoveryClient
public class AddressManagementServiceApplication {
public static void main(String[] args) {
SpringApplication.run(AddressManagementServiceApplication.class, args);
}
}
@RestController
@RequestMapping("/api/address")
public class AddressController {
@Autowired
private AddressService addressService;
@GetMapping("/{id}")
public ResponseEntity<AddressDto> getAddressById(@PathVariable Long id) {
AddressDto address = addressService.getAddressById(id);
return ResponseEntity.ok(address);
}
@PostMapping
public ResponseEntity<AddressDto> createAddress(@RequestBody AddressDto addressDto) {
AddressDto createdAddress = addressService.createAddress(addressDto);
return ResponseEntity.status(HttpStatus.CREATED).body(createdAddress);
}
@PutMapping("/{id}")
public ResponseEntity<AddressDto> updateAddress(@PathVariable Long id, @RequestBody AddressDto addressDto) {
AddressDto updatedAddress = addressService.updateAddress(id, addressDto);
return ResponseEntity.ok(updatedAddress);
}
@DeleteMapping("/{id}")
public ResponseEntity<Void> deleteAddress(@PathVariable Long id) {
addressService.deleteAddress(id);
return ResponseEntity.noContent().build();
}
}
```
#### 2. 地名业务审批管理服务
```java
@SpringBootApplication
@EnableDiscoveryClient
public class ApprovalManagementServiceApplication {
public static void main(String[] args) {
SpringApplication.run(ApprovalManagementServiceApplication.class, args);
}
}
@RestController
@RequestMapping("/api/approval")
public class ApprovalController {
@Autowired
private ApprovalService approvalService;
@GetMapping("/{id}")
public ResponseEntity<ApprovalDto> getApprovalById(@PathVariable Long id) {
ApprovalDto approval = approvalService.getApprovalById(id);
return ResponseEntity.ok(approval);
}
@PostMapping
public ResponseEntity<ApprovalDto> createApproval(@RequestBody ApprovalDto approvalDto) {
ApprovalDto createdApproval = approvalService.createApproval(approvalDto);
return ResponseEntity.status(HttpStatus.CREATED).body(createdApproval);
}
@PutMapping("/{id}")
public ResponseEntity<ApprovalDto> updateApproval(@PathVariable Long id, @RequestBody ApprovalDto approvalDto) {
ApprovalDto updatedApproval = approvalService.updateApproval(id, approvalDto);
return ResponseEntity.ok(updatedApproval);
}
@DeleteMapping("/{id}")
public ResponseEntity<Void> deleteApproval(@PathVariable Long id) {
approvalService.deleteApproval(id);
return ResponseEntity.noContent().build();
}
}
```
#### 3. 移动服务(对接玛依办APP)
```java
@SpringBootApplication
@EnableDiscoveryClient
public class MobileServiceApplication {
public static void main(String[] args) {
SpringApplication.run(MobileServiceApplication.class, args);
}
}
@RestController
@RequestMapping("/api/mobile")
public class MobileController {
@Autowired
private MobileService mobileService;
@GetMapping("/address/{id}")
public ResponseEntity<AddressDto> getAddressById(@PathVariable Long id) {
AddressDto address = mobileService.getAddressById(id);
return ResponseEntity.ok(address);
}
@PostMapping("/address")
public ResponseEntity<AddressDto> createAddress(@RequestBody AddressDto addressDto) {
AddressDto createdAddress = mobileService.createAddress(addressDto);
return ResponseEntity.status(HttpStatus.CREATED).body(createdAddress);
}
}
```
### 微服务治理
为了确保微服务之间的高效协同和可靠性,你需要实现以下服务治理措施:
1. **节点管理**:
- 使用注册中心(如Eureka、zookeeper、nacos)进行服务注册和发现。
- 实现心跳检测和节点摘除机制。
2. **负载均衡**:
- 使用Spring Cloud Ribbon或Netflix Zuul实现负载均衡。
3. **服务路由**:
- 使用条件表达式或正则表达式配置服务路由。
4. **服务容错**:
- 使用Hystrix实现限流熔断。
5. **限流熔断**:
- 使用Resilience4j或Sentinel实现限流和熔断。
通过以上示例和服务治理措施,你可以构建一个健壮、可靠的微服务架构,满足克拉玛依市地名地址数据库服务平台的需求。
阅读全文
相关推荐
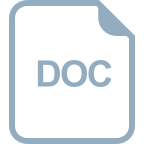
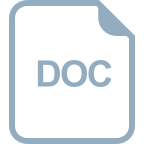
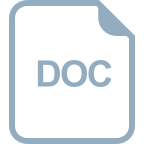
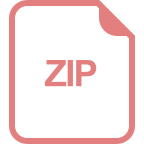
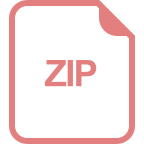
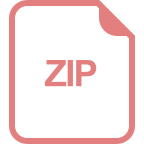
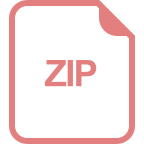
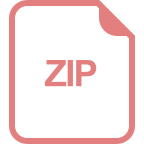
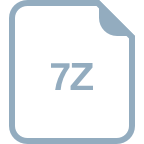
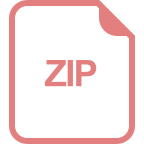
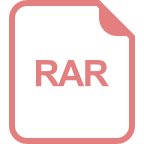
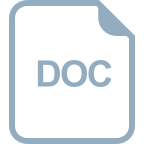
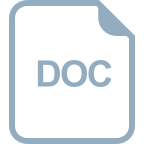
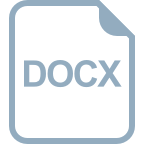
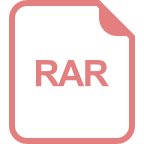