c++创建thread
时间: 2023-08-29 21:12:04 浏览: 88
在C++中,创建线程的方式有多种方法。一种常见的方式是使用函数指针来创建线程。可以使用std::thread类来创建线程对象,并将函数指针作为参数传递给std::thread的构造函数。例如,可以使用以下代码创建一个线程对象并开启一个线程:
```
#include <cstdio>
#include <thread>
void fun(int a) {
printf("%d\n", a);
}
int main() {
int a = 1;
std::thread t(fun, a);
t.join();
return 0;
}
```
另一种方式是使用函数对象来创建线程。可以定义一个类,并重载该类的operator()()运算符,将该类的对象作为参数传递给std::thread的构造函数。例如,可以使用以下代码创建一个线程对象并开启一个线程:
```
#include <iostream>
#include <thread>
#include <chrono>
using namespace std;
class Thread_Test {
public:
void operator()() {
for (int i = 0; i < 10; i++) {
cout << "Thread_Test is running..." << endl;
std::this_thread::sleep_for(std::chrono::seconds(1));
}
}
};
int main() {
thread threadobj((Thread_Test()));
cout << "main Thread is running..." << endl;
threadobj.join();
cout << "exit from main Thread" << endl;
return 0;
}
```
此外,还可以使用lambda函数来创建线程。可以定义一个lambda函数,并将其作为参数传递给std::thread的构造函数。例如,可以使用以下代码创建一个线程对象并开启一个线程:
```
#include <iostream>
#include <thread>
int main() {
int a = 1;
std::thread t([&a]() {
std::cout << a << std::endl;
});
t.join();
return 0;
}
```
总结起来,C++中创建线程的方式包括使用函数指针、函数对象和lambda函数。具体选择哪种方式取决于实际需求和个人偏好。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *3* [C++创建线程的三种方式](https://blog.csdn.net/yangcunbiao/article/details/131151630)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *2* [c++ 学习之 多线程(一) thread对象的创建](https://blog.csdn.net/weixin_45074185/article/details/104363741)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
阅读全文
相关推荐
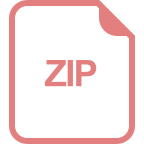














