uniapp父组件传如何调用子组件的方法除了使用ref还可以怎么调用子组件中的方法
时间: 2023-11-29 22:42:34 浏览: 155
除了使用ref,还可以通过事件派发的方式调用子组件中的方法。具体实现方式如下:
1. 在子组件中定义一个方法,例如:
```
methods: {
childMethod() {
console.log('子组件方法被调用');
}
}
```
2. 在子组件中定义一个事件,例如:
```
mounted() {
this.$emit('childEvent', this.childMethod);
}
```
3. 在父组件中通过监听子组件的事件来获取子组件中的方法,例如:
```
<template>
<div>
<child-component @childEvent="onChildEvent"></child-component>
</div>
</template>
<script>
export default {
methods: {
onChildEvent(childMethod) {
childMethod();
}
}
}
</script>
```
这样就可以在父组件中调用子组件中的方法了。
相关问题
uniapp中父组件怎么调用子组件的方法
在uni-app中,父组件可以通过使用ref属性来获取子组件的实例,并调用子组件的方法。下面是一个示例:
1. 在子组件中,给子组件的标签添加ref属性,例如:
```
<template>
<div ref="childComponent">
<!-- 子组件内容 -->
</div>
</template>
```
2. 在父组件中,使用this.$refs来获取子组件的实例,并调用子组件的方法,例如:
```
<template>
<div>
<child-component ref="myChildComponent"></child-component>
<button @click="callChildMethod">调用子组件方法</button>
</div>
</template>
<script>
export default {
methods: {
callChildMethod() {
this.$refs.myChildComponent.childMethod();
}
}
}
</script>
```
在上面的示例中,父组件通过ref属性给子组件标签命名为"myChildComponent",然后在父组件的方法中使用this.$refs.myChildComponent来获取子组件的实例,并调用子组件的方法childMethod()。
注意:在使用ref属性获取子组件实例之前,需要确保子组件已经被渲染到父组件中。可以使用v-if或者v-show来控制子组件的显示与隐藏,以确保在调用子组件方法时子组件已经存在。
uniapp父组件调用子组件的方法
在uni-app中,父组件可以调用子组件的方法。首先,确保已经在父组件中引入了子组件。然后,可以通过`ref`属性给子组件添加一个引用,然后通过这个引用来调用子组件的方法。
下面是一个示例:
在父组件中:
```html
<template>
<div>
<child ref="childRef"></child>
<button @click="callChildMethod">调用子组件方法</button>
</div>
</template>
<script>
import child from '@/components/child.vue';
export default {
components: {
child,
},
methods: {
callChildMethod() {
this.$refs.childRef.childMethod(); // 调用子组件的方法
},
},
};
</script>
```
在子组件中(child.vue):
```html
<template>
<div>
子组件内容
</div>
</template>
<script>
export default {
methods: {
childMethod() {
console.log('子组件方法被调用');
},
},
};
</script>
```
在父组件中,使用`ref`给子组件添加一个引用,然后通过`this.$refs.childRef`来获取子组件的实例,进而调用子组件的方法。
注意:使用`ref`引用子组件时,需要确保子组件已经被渲染到父组件中。
相关推荐
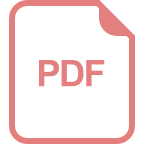
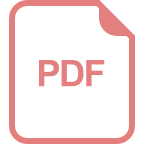
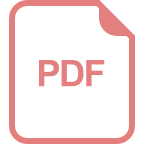












