用贪心写国际象棋 python
时间: 2024-12-23 11:10:31 浏览: 4
在Python中使用贪心算法来设计一个简单的国际象棋游戏并非最佳选择,因为贪心算法通常适用于每一步都能局部最优的问题,而国际象棋涉及到复杂的策略、搜索树和规则判断,更适合使用博弈论中的搜索算法如Alpha-Beta剪枝、Minimax或更先进的AI技术(如MCTS)。
然而,如果你想通过贪心策略实现一些基础的棋盘操作,比如最小步数移动棋子到目标位置,你可以这样做:
```python
def greedy_chess_move(board, start, end):
# 简单的“最短路径”贪心处理
path = []
current = start
while current != end:
if board[current] == None: # 空位可以直接走
path.append(current)
current = end
else:
directions = [(0, 1), (0, -1), (1, 0), (-1, 0)] # 四个方向
for dx, dy in directions:
next_pos = (current[0] + dx, current[1] + dy)
if 0 <= next_pos[0] < len(board) and 0 <= next_pos[1] < len(board[0]) and board[next_pos] == None:
path.append(next_pos)
current = next_pos
break
return path
# 示例
board = [
[None, None, 'P', None],
['R', 'N', 'B', 'Q'],
[None, None, None, None]
]
start = (0, 0) # 起始位置
end = (2, 1) # 目标位置
path = greedy_chess_move(board, start, end)
print(f"从{start}到{end}的简单贪心路径:{path}")
```
这个例子仅考虑了直线上方、下方、左方和右方的空格作为合法走法,并不是完整的国际象棋规则。实际的国际象棋AI需要更复杂的设计和深度学习。
阅读全文
相关推荐
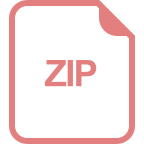

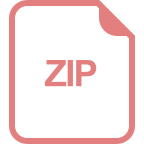
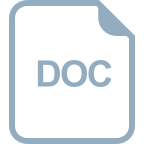
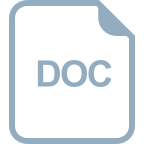
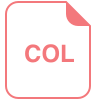
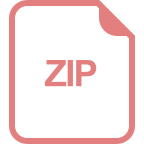
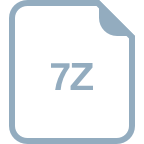
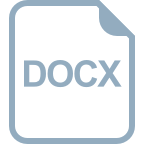
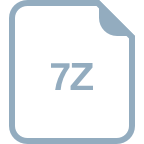
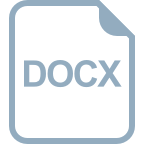