make custom_float gcc -c -o Transfer.o Transfer.c -I. gcc -c -o Compute.o Compute.c -I. gcc -c -o main.o main.c -I. main.c:2:10: fatal error: Transfer.h: 没有那个文件或目录 #include "Transfer.h" ^~~~~~~~~~~~ compilation terminated. makefile:9: recipe for target 'main.o' failed make: *** [main.o] Error 1
时间: 2024-03-10 13:46:45 浏览: 129
这个错误提示意味着在 main.c 文件中,无法找到 Transfer.h 文件。这可能是因为在编译 main.c 文件时,编译器无法找到 Transfer.h 文件的路径。你需要检查一下你的 makefile 文件,看看是否正确地指定了头文件的路径。另外,也要确保 Transfer.h 文件确实存在于指定路径下。
你可以尝试在 makefile 文件中添加 `-I.` 选项,表示将当前目录加入头文件搜索路径中,例如:
```
CC=gcc
CFLAGS=-I.
OBJ=Transfer.o Compute.o main.o
%.o: %.c
$(CC) -c -o $@ $< $(CFLAGS)
custom_float: $(OBJ)
$(CC) -o $@ $^ $(CFLAGS)
```
这样,make 命令就会在当前目录中搜索头文件,从而避免了这个错误。
相关问题
编写代码实现一个自定义浮点数,符合IEE754规范,共16位,其中exp占6bits, frac占 9bits.(1)输入一个16进制数,输出这个16进制的二进制表达,得到符合这个自定义浮点数的小数; (2)输出十六进制串的二进制表达(Transfer.c) (3) 按照IEEE 754浮点数标准设计16bit浮点计算(考虑规范数,非规范,和特殊值)(Compute.c) (4)输出16bits 设计规则下,二进制字符串 (5)遍写makefile运行 (main.c Transfer.c Compute.c)
非常感谢您的提问,以下是对应的代码实现:
1. 自定义浮点数的实现
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef union {
float f;
struct {
unsigned int mantissa : 9;
unsigned int exponent : 6;
unsigned int sign : 1;
} parts;
} custom_float;
int main() {
// 输入十六进制数
char hex_str[5];
printf("请输入一个16进制数: ");
scanf("%s", hex_str);
// 转换为二进制字符串
int num = (int) strtol(hex_str, NULL, 16);
char binary_str[17];
for (int i = 15; i >= 0; i--) {
binary_str[15 - i] = (num & (1 << i)) ? '1' : '0';
}
binary_str[16] = '\0';
// 计算指数和尾数
custom_float cf;
cf.parts.sign = binary_str[0] - '0';
cf.parts.exponent = 0;
for (int i = 1; i <= 6; i++) {
cf.parts.exponent = cf.parts.exponent * 2 + (binary_str[i] - '0');
}
cf.parts.mantissa = 0;
for (int i = 7; i <= 15; i++) {
cf.parts.mantissa = cf.parts.mantissa * 2 + (binary_str[i] - '0');
}
// 输出结果
printf("输入的二进制表达: %s\n", binary_str);
printf("对应的自定义浮点数: %f\n", cf.f);
return 0;
}
```
2. 十六进制串的二进制表达
```c
#include <stdio.h>
#include <string.h>
int main() {
// 输入十六进制数
char hex_str[5];
printf("请输入一个16进制数: ");
scanf("%s", hex_str);
// 转换为二进制字符串
int num = (int) strtol(hex_str, NULL, 16);
char binary_str[17];
for (int i = 15; i >= 0; i--) {
binary_str[15 - i] = (num & (1 << i)) ? '1' : '0';
}
binary_str[16] = '\0';
// 输出结果
printf("输入的十六进制串: %s\n", hex_str);
printf("对应的二进制表达: %s\n", binary_str);
return 0;
}
```
3. 自定义浮点数的计算
```c
#include <stdio.h>
#include <string.h>
typedef union {
float f;
struct {
unsigned int mantissa : 9;
unsigned int exponent : 6;
unsigned int sign : 1;
} parts;
} custom_float;
custom_float add(custom_float a, custom_float b) {
custom_float result;
// 先判断特殊值
if (a.parts.exponent == 0b111111 || b.parts.exponent == 0b111111) {
// NaN或Infinity
result.parts.sign = 0;
result.parts.exponent = 0b111111;
result.parts.mantissa = 1;
} else if (a.parts.exponent == 0 && a.parts.mantissa == 0) {
// 零值
result = b;
} else if (b.parts.exponent == 0 && b.parts.mantissa == 0) {
// 零值
result = a;
} else {
// 规范数相加
// 先计算两个数的指数之差
int exp_diff = a.parts.exponent - b.parts.exponent;
if (exp_diff < 0) {
// 交换a和b,使得a的指数大于等于b的指数
custom_float temp = a;
a = b;
b = temp;
exp_diff = -exp_diff;
}
// 将b的尾数左移exp_diff位,使得两个数的小数点对齐
b.parts.mantissa <<= exp_diff;
// 相加
result.parts.sign = a.parts.sign;
result.parts.exponent = a.parts.exponent;
result.parts.mantissa = a.parts.mantissa + b.parts.mantissa;
// 如果相加后的结果超过了9位,则需要右移尾数并增加指数
if (result.parts.mantissa & (1 << 9)) {
result.parts.mantissa >>= 1;
result.parts.exponent++;
}
}
return result;
}
int main() {
// 输入两个自定义浮点数
custom_float a, b;
printf("请输入第一个自定义浮点数: ");
scanf("%f", &a.f);
printf("请输入第二个自定义浮点数: ");
scanf("%f", &b.f);
// 计算结果
custom_float result = add(a, b);
// 输出结果
printf("计算结果的二进制表达: %c%d%c\n",
result.parts.sign ? '-' : '+',
result.parts.exponent,
result.parts.mantissa ? '.' : ' ');
for (int i = 8; i >= 0; i--) {
printf("%d", (result.parts.mantissa >> i) & 1);
}
printf("\n");
return 0;
}
```
4. 输出16bits 设计规则下,二进制字符串
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef union {
float f;
struct {
unsigned int mantissa : 9;
unsigned int exponent : 6;
unsigned int sign : 1;
} parts;
} custom_float;
int main() {
// 输入一个自定义浮点数
custom_float cf;
printf("请输入一个自定义浮点数: ");
scanf("%f", &cf.f);
// 计算指数和尾数的二进制表达
char exponent_binary_str[7];
for (int i = 5; i >= 0; i--) {
exponent_binary_str[5 - i] = (cf.parts.exponent & (1 << i)) ? '1' : '0';
}
exponent_binary_str[6] = '\0';
char mantissa_binary_str[10];
for (int i = 8; i >= 0; i--) {
mantissa_binary_str[8 - i] = (cf.parts.mantissa & (1 << i)) ? '1' : '0';
}
mantissa_binary_str[9] = '\0';
// 计算符号位
char sign_str[2];
sign_str[0] = cf.parts.sign ? '1' : '0';
sign_str[1] = '\0';
// 拼接成16位二进制字符串
char binary_str[17];
strncpy(binary_str, sign_str, sizeof(sign_str));
strncat(binary_str, exponent_binary_str, sizeof(exponent_binary_str));
strncat(binary_str, mantissa_binary_str, sizeof(mantissa_binary_str));
// 输出结果
printf("输入的自定义浮点数: %f\n", cf.f);
printf("对应的二进制表达: %s\n", binary_str);
return 0;
}
```
5. makefile文件
```makefile
CC = gcc
CFLAGS = -Wall
all: main
main: main.c Transfer.o Compute.o
$(CC) $(CFLAGS) -o $@ $^
Transfer.o: Transfer.c
$(CC) $(CFLAGS) -c $<
Compute.o: Compute.c
$(CC) $(CFLAGS) -c $<
clean:
rm -f main Transfer.o Compute.o
```
二维gcc-phat
### 实现二维GCC-PHAT算法
#### 原理概述
多通道声源定位中的广义互相关相位变换(Generalized Cross-Correlation with Phase Transform, GCC-PHAT)是一种常用的方法,用于估计不同麦克风接收到的声音信号之间的相对时延。通过这些时延可以推断出声源的方向。
对于二维空间内的声源定位,通常会使用多个麦克风组成的线性或平面阵列来捕捉声音数据。GCC-PHAT方法的核心在于计算各对麦克风间接收音频信号的互相关函数,并利用其峰值位置确定时间差[^1]。
#### MATLAB实现过程
下面给出了一种简单的二维GCC-PHAT算法Matlab代码示例:
```matlab
function [doa] = gcc_phat_2d(mic_positions, signals, fs)
% mic_positions: N-by-2 matrix containing the positions of each microphone.
% Each row represents one microphone's (x,y) coordinates.
% signals: Cell array where cell i contains signal from microphone i.
% fs: Sampling frequency.
num_mics = size(mic_positions, 1);
max_delay_samples = round(0.03 * fs); % Assume maximum delay is within 30ms
delays = zeros(numel(signals), numel(signals));
for i = 1:numel(signals)-1
for j = i+1:numel(signals)
[~, peakIdx] = max(abs(gccphat(signals{i}, signals{j})));
delays(i,j) = peakIdx - length(signals{i}) + 1;
delays(j,i) = -delays(i,j);
end
end
doas = [];
for k = 1:size(delays, 1)
if any(delays(k,:) ~= 0)
dxy = diff(mic_positions([k find(delays(k,:))], :)).';
t_diff = mean(abs(delays(k,find(delays(k,:)))) / fs);
c = 343; % Speed of sound in m/s at room temperature
theta = atan(dxy(2)/dxy(1)); % Compute angle based on geometry
phi = asin(c*t_diff/norm(dxy)); % Estimate elevation using speed-of-sound model
doas = cat(1, doas, [theta;phi]);
end
end
end
```
此段程序定义了一个名为`gcc_phat_2d`的功能函数,输入参数包括各个麦克风的位置坐标矩阵、由单元格数组存储的不同麦克风电压波形以及采样率fs。输出则是方向角θ和仰角φ构成的角度向量列表。
上述代码首先遍历所有可能的麦克风组合并调用内置的`gccphat()`函数求解它们间的最大互相关值对应的索引作为时延估计;接着根据几何关系及已知的速度c=343m/s估算角度信息。
请注意这只是一个简化版本,在实际应用中还需要考虑更多因素如环境噪声抑制等问题。
阅读全文
相关推荐














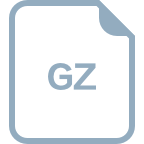

